Twitter a Social Media Scrape#
What is the Twitterverse Discussing?#
# The Yahoo! Where On Earth ID for the entire world is 1.
# See https://dev.twitter.com/docs/api/1.1/get/trends/place and
# http://developer.yahoo.com/geo/geoplanet/
WORLD_WOE_ID = 1
US_WOE_ID = 23424977
# Prefix ID with the underscore for query string parameterization.
# Without the underscore, the twitter package appends the ID value
# to the URL itself as a special case keyword argument.
world_trends = mathcs_twitter_api.trends.place(_id=WORLD_WOE_ID)
us_trends = mathcs_twitter_api.trends.place(_id=US_WOE_ID)
for i in range(10):
print(world_trends[0]['trends'][i]['query'])
%23MelodicVoiceINDay
%23%EC%9D%B4%EC%97%94%EC%9D%B4_%EB%AF%B8%EC%86%8C%EA%B0%80_%EC%84%B8%EC%83%81%EC%9D%84_%EB%B0%9D%ED%9E%8C%EB%82%A0
%23HappyINDay
%22ExtraordINary+DAY%22
%22Jason+Roy%22
%23QGvsLQ
Euphoria
%22LET+SOMEBODY+GO+MUSIC+VIDEO%22
%23%D8%AD%D9%84%D9%85_%D8%A7%D9%84%D9%86%D9%87%D8%A7%D9%8A%D9%8A_%D9%8A%D8%A7_%D8%A7%D9%87%D9%84%D9%8A
%22Wordle+234%22
for i in range(3):
print(us_trends[0]['trends'][i]['query'])
%23MondayMotivation
%22Wordle+233+X%22
%22Pusha+T%22
Here is a nicer display of the data using json
package. JSON is anotherway to store data and is utilized in many no-sql databases.
import json
print(json.dumps(world_trends, indent=1))
print(json.dumps(us_trends, indent=1))
[
{
"trends": [
{
"name": "#MelodicVoiceINDay",
"url": "http://twitter.com/search?q=%23MelodicVoiceINDay",
"promoted_content": null,
"query": "%23MelodicVoiceINDay",
"tweet_volume": 268594
},
{
"name": "#\uc774\uc5d4\uc774_\ubbf8\uc18c\uac00_\uc138\uc0c1\uc744_\ubc1d\ud78c\ub0a0",
"url": "http://twitter.com/search?q=%23%EC%9D%B4%EC%97%94%EC%9D%B4_%EB%AF%B8%EC%86%8C%EA%B0%80_%EC%84%B8%EC%83%81%EC%9D%84_%EB%B0%9D%ED%9E%8C%EB%82%A0",
"promoted_content": null,
"query": "%23%EC%9D%B4%EC%97%94%EC%9D%B4_%EB%AF%B8%EC%86%8C%EA%B0%80_%EC%84%B8%EC%83%81%EC%9D%84_%EB%B0%9D%ED%9E%8C%EB%82%A0",
"tweet_volume": 238069
},
{
"name": "#HappyINDay",
"url": "http://twitter.com/search?q=%23HappyINDay",
"promoted_content": null,
"query": "%23HappyINDay",
"tweet_volume": 355991
},
{
"name": "ExtraordINary DAY",
"url": "http://twitter.com/search?q=%22ExtraordINary+DAY%22",
"promoted_content": null,
"query": "%22ExtraordINary+DAY%22",
"tweet_volume": 190416
},
{
"name": "Jason Roy",
"url": "http://twitter.com/search?q=%22Jason+Roy%22",
"promoted_content": null,
"query": "%22Jason+Roy%22",
"tweet_volume": 12589
},
{
"name": "#QGvsLQ",
"url": "http://twitter.com/search?q=%23QGvsLQ",
"promoted_content": null,
"query": "%23QGvsLQ",
"tweet_volume": null
},
{
"name": "Euphoria",
"url": "http://twitter.com/search?q=Euphoria",
"promoted_content": null,
"query": "Euphoria",
"tweet_volume": 1744336
},
{
"name": "LET SOMEBODY GO MUSIC VIDEO",
"url": "http://twitter.com/search?q=%22LET+SOMEBODY+GO+MUSIC+VIDEO%22",
"promoted_content": null,
"query": "%22LET+SOMEBODY+GO+MUSIC+VIDEO%22",
"tweet_volume": 25975
},
{
"name": "#\u062d\u0644\u0645_\u0627\u0644\u0646\u0647\u0627\u064a\u064a_\u064a\u0627_\u0627\u0647\u0644\u064a",
"url": "http://twitter.com/search?q=%23%D8%AD%D9%84%D9%85_%D8%A7%D9%84%D9%86%D9%87%D8%A7%D9%8A%D9%8A_%D9%8A%D8%A7_%D8%A7%D9%87%D9%84%D9%8A",
"promoted_content": null,
"query": "%23%D8%AD%D9%84%D9%85_%D8%A7%D9%84%D9%86%D9%87%D8%A7%D9%8A%D9%8A_%D9%8A%D8%A7_%D8%A7%D9%87%D9%84%D9%8A",
"tweet_volume": 145044
},
{
"name": "Wordle 234",
"url": "http://twitter.com/search?q=%22Wordle+234%22",
"promoted_content": null,
"query": "%22Wordle+234%22",
"tweet_volume": 31501
},
{
"name": "Motorine 80",
"url": "http://twitter.com/search?q=%22Motorine+80%22",
"promoted_content": null,
"query": "%22Motorine+80%22",
"tweet_volume": 14989
},
{
"name": "Poutine",
"url": "http://twitter.com/search?q=Poutine",
"promoted_content": null,
"query": "Poutine",
"tweet_volume": 26576
},
{
"name": "Sis Tamara",
"url": "http://twitter.com/search?q=%22Sis+Tamara%22",
"promoted_content": null,
"query": "%22Sis+Tamara%22",
"tweet_volume": null
},
{
"name": "\u30c7\u30b8\u30bf\u30ebKFC\u30ab\u30fc\u30c91\u4e07\u5186\u5206\u30d7\u30ec\u30bc\u30f3\u30c8",
"url": "http://twitter.com/search?q=%E3%83%87%E3%82%B8%E3%82%BF%E3%83%ABKFC%E3%82%AB%E3%83%BC%E3%83%891%E4%B8%87%E5%86%86%E5%88%86%E3%83%97%E3%83%AC%E3%82%BC%E3%83%B3%E3%83%88",
"promoted_content": null,
"query": "%E3%83%87%E3%82%B8%E3%82%BF%E3%83%ABKFC%E3%82%AB%E3%83%BC%E3%83%891%E4%B8%87%E5%86%86%E5%88%86%E3%83%97%E3%83%AC%E3%82%BC%E3%83%B3%E3%83%88",
"tweet_volume": null
},
{
"name": "\u6295\u7a3f\u306e\u4e2d",
"url": "http://twitter.com/search?q=%E6%8A%95%E7%A8%BF%E3%81%AE%E4%B8%AD",
"promoted_content": null,
"query": "%E6%8A%95%E7%A8%BF%E3%81%AE%E4%B8%AD",
"tweet_volume": 152648
},
{
"name": "OFF\u30d1\u30c3\u30af",
"url": "http://twitter.com/search?q=OFF%E3%83%91%E3%83%83%E3%82%AF",
"promoted_content": null,
"query": "OFF%E3%83%91%E3%83%83%E3%82%AF",
"tweet_volume": null
},
{
"name": "Pusha T",
"url": "http://twitter.com/search?q=%22Pusha+T%22",
"promoted_content": null,
"query": "%22Pusha+T%22",
"tweet_volume": 11900
},
{
"name": "Vyno",
"url": "http://twitter.com/search?q=Vyno",
"promoted_content": null,
"query": "Vyno",
"tweet_volume": null
},
{
"name": "Jules",
"url": "http://twitter.com/search?q=Jules",
"promoted_content": null,
"query": "Jules",
"tweet_volume": 281255
},
{
"name": "Hakl\u0131y\u0131zKazanaca\u011f\u0131z",
"url": "http://twitter.com/search?q=Hakl%C4%B1y%C4%B1zKazanaca%C4%9F%C4%B1z",
"promoted_content": null,
"query": "Hakl%C4%B1y%C4%B1zKazanaca%C4%9F%C4%B1z",
"tweet_volume": 21512
},
{
"name": "Yoli",
"url": "http://twitter.com/search?q=Yoli",
"promoted_content": null,
"query": "Yoli",
"tweet_volume": 11235
},
{
"name": "B\u0130Z ONA RE\u0130S DED\u0130K",
"url": "http://twitter.com/search?q=%22B%C4%B0Z+ONA+RE%C4%B0S+DED%C4%B0K%22",
"promoted_content": null,
"query": "%22B%C4%B0Z+ONA+RE%C4%B0S+DED%C4%B0K%22",
"tweet_volume": null
},
{
"name": "Coldplay",
"url": "http://twitter.com/search?q=Coldplay",
"promoted_content": null,
"query": "Coldplay",
"tweet_volume": 36236
},
{
"name": "Russell Brand",
"url": "http://twitter.com/search?q=%22Russell+Brand%22",
"promoted_content": null,
"query": "%22Russell+Brand%22",
"tweet_volume": 17595
},
{
"name": "S\u00fcle",
"url": "http://twitter.com/search?q=S%C3%BCle",
"promoted_content": null,
"query": "S%C3%BCle",
"tweet_volume": 32025
},
{
"name": "Tulsi Gabbard",
"url": "http://twitter.com/search?q=%22Tulsi+Gabbard%22",
"promoted_content": null,
"query": "%22Tulsi+Gabbard%22",
"tweet_volume": null
},
{
"name": "Quetta",
"url": "http://twitter.com/search?q=Quetta",
"promoted_content": null,
"query": "Quetta",
"tweet_volume": null
},
{
"name": "Fabr\u00edcio Bruno",
"url": "http://twitter.com/search?q=%22Fabr%C3%ADcio+Bruno%22",
"promoted_content": null,
"query": "%22Fabr%C3%ADcio+Bruno%22",
"tweet_volume": null
},
{
"name": "BOLSONARO INEPTO",
"url": "http://twitter.com/search?q=%22BOLSONARO+INEPTO%22",
"promoted_content": null,
"query": "%22BOLSONARO+INEPTO%22",
"tweet_volume": null
},
{
"name": "Piers Corbyn",
"url": "http://twitter.com/search?q=%22Piers+Corbyn%22",
"promoted_content": null,
"query": "%22Piers+Corbyn%22",
"tweet_volume": null
},
{
"name": "\u7b2c18\u56de",
"url": "http://twitter.com/search?q=%E7%AC%AC18%E5%9B%9E",
"promoted_content": null,
"query": "%E7%AC%AC18%E5%9B%9E",
"tweet_volume": null
},
{
"name": "Lost Ark",
"url": "http://twitter.com/search?q=%22Lost+Ark%22",
"promoted_content": null,
"query": "%22Lost+Ark%22",
"tweet_volume": 13471
},
{
"name": "Saltong 26",
"url": "http://twitter.com/search?q=%22Saltong+26%22",
"promoted_content": null,
"query": "%22Saltong+26%22",
"tweet_volume": null
},
{
"name": "mar-a-lago",
"url": "http://twitter.com/search?q=mar-a-lago",
"promoted_content": null,
"query": "mar-a-lago",
"tweet_volume": 57425
},
{
"name": "Razzies",
"url": "http://twitter.com/search?q=Razzies",
"promoted_content": null,
"query": "Razzies",
"tweet_volume": null
},
{
"name": "87 \u00c9 DO SPORT",
"url": "http://twitter.com/search?q=%2287+%C3%89+DO+SPORT%22",
"promoted_content": null,
"query": "%2287+%C3%89+DO+SPORT%22",
"tweet_volume": 12174
},
{
"name": "Cau\u00ea Moura",
"url": "http://twitter.com/search?q=%22Cau%C3%AA+Moura%22",
"promoted_content": null,
"query": "%22Cau%C3%AA+Moura%22",
"tweet_volume": null
},
{
"name": "HAPPY BIRTHDAY YUNHYEONG",
"url": "http://twitter.com/search?q=%22HAPPY+BIRTHDAY+YUNHYEONG%22",
"promoted_content": null,
"query": "%22HAPPY+BIRTHDAY+YUNHYEONG%22",
"tweet_volume": 40745
},
{
"name": "National Archives",
"url": "http://twitter.com/search?q=%22National+Archives%22",
"promoted_content": null,
"query": "%22National+Archives%22",
"tweet_volume": 40995
},
{
"name": "Hermann Tertsch",
"url": "http://twitter.com/search?q=%22Hermann+Tertsch%22",
"promoted_content": null,
"query": "%22Hermann+Tertsch%22",
"tweet_volume": null
},
{
"name": "Frontier",
"url": "http://twitter.com/search?q=Frontier",
"promoted_content": null,
"query": "Frontier",
"tweet_volume": 21842
},
{
"name": "Nawaz",
"url": "http://twitter.com/search?q=Nawaz",
"promoted_content": null,
"query": "Nawaz",
"tweet_volume": 10075
},
{
"name": "KPMG",
"url": "http://twitter.com/search?q=KPMG",
"promoted_content": null,
"query": "KPMG",
"tweet_volume": 12431
},
{
"name": "Lovie",
"url": "http://twitter.com/search?q=Lovie",
"promoted_content": null,
"query": "Lovie",
"tweet_volume": 20737
},
{
"name": "Acacia",
"url": "http://twitter.com/search?q=Acacia",
"promoted_content": null,
"query": "Acacia",
"tweet_volume": null
},
{
"name": "Cheaper by the Dozen",
"url": "http://twitter.com/search?q=%22Cheaper+by+the+Dozen%22",
"promoted_content": null,
"query": "%22Cheaper+by+the+Dozen%22",
"tweet_volume": null
},
{
"name": "Thibs",
"url": "http://twitter.com/search?q=Thibs",
"promoted_content": null,
"query": "Thibs",
"tweet_volume": null
},
{
"name": "\uc1fc\ud2b8\ud2b8\ub799",
"url": "http://twitter.com/search?q=%EC%87%BC%ED%8A%B8%ED%8A%B8%EB%9E%99",
"promoted_content": null,
"query": "%EC%87%BC%ED%8A%B8%ED%8A%B8%EB%9E%99",
"tweet_volume": 372283
},
{
"name": "Sam Harris",
"url": "http://twitter.com/search?q=%22Sam+Harris%22",
"promoted_content": null,
"query": "%22Sam+Harris%22",
"tweet_volume": null
},
{
"name": "#LQvQG",
"url": "http://twitter.com/search?q=%23LQvQG",
"promoted_content": null,
"query": "%23LQvQG",
"tweet_volume": null
}
],
"as_of": "2022-02-07T19:14:53Z",
"created_at": "2022-02-06T09:59:48Z",
"locations": [
{
"name": "Worldwide",
"woeid": 1
}
]
}
]
[
{
"trends": [
{
"name": "#MondayMotivation",
"url": "http://twitter.com/search?q=%23MondayMotivation",
"promoted_content": null,
"query": "%23MondayMotivation",
"tweet_volume": 133193
},
{
"name": "Wordle 233 X",
"url": "http://twitter.com/search?q=%22Wordle+233+X%22",
"promoted_content": null,
"query": "%22Wordle+233+X%22",
"tweet_volume": 12128
},
{
"name": "Pusha T",
"url": "http://twitter.com/search?q=%22Pusha+T%22",
"promoted_content": null,
"query": "%22Pusha+T%22",
"tweet_volume": 11870
},
{
"name": "#SignsYouMightBeAnAdult",
"url": "http://twitter.com/search?q=%23SignsYouMightBeAnAdult",
"promoted_content": null,
"query": "%23SignsYouMightBeAnAdult",
"tweet_volume": null
},
{
"name": "Tulsi Gabbard",
"url": "http://twitter.com/search?q=%22Tulsi+Gabbard%22",
"promoted_content": null,
"query": "%22Tulsi+Gabbard%22",
"tweet_volume": null
},
{
"name": "Frontier",
"url": "http://twitter.com/search?q=Frontier",
"promoted_content": null,
"query": "Frontier",
"tweet_volume": 21842
},
{
"name": "National Archives",
"url": "http://twitter.com/search?q=%22National+Archives%22",
"promoted_content": null,
"query": "%22National+Archives%22",
"tweet_volume": 40995
},
{
"name": "Mar-a-lago",
"url": "http://twitter.com/search?q=Mar-a-lago",
"promoted_content": null,
"query": "Mar-a-lago",
"tweet_volume": 57425
},
{
"name": "Razzies",
"url": "http://twitter.com/search?q=Razzies",
"promoted_content": null,
"query": "Razzies",
"tweet_volume": null
},
{
"name": "#MoviesThatNeedGlasses",
"url": "http://twitter.com/search?q=%23MoviesThatNeedGlasses",
"promoted_content": null,
"query": "%23MoviesThatNeedGlasses",
"tweet_volume": null
},
{
"name": "Russell Brand",
"url": "http://twitter.com/search?q=%22Russell+Brand%22",
"promoted_content": null,
"query": "%22Russell+Brand%22",
"tweet_volume": 17595
},
{
"name": "Thibs",
"url": "http://twitter.com/search?q=Thibs",
"promoted_content": null,
"query": "Thibs",
"tweet_volume": null
},
{
"name": "#MelodicVoiceINDay",
"url": "http://twitter.com/search?q=%23MelodicVoiceINDay",
"promoted_content": null,
"query": "%23MelodicVoiceINDay",
"tweet_volume": 268594
},
{
"name": "Cheaper by the Dozen",
"url": "http://twitter.com/search?q=%22Cheaper+by+the+Dozen%22",
"promoted_content": null,
"query": "%22Cheaper+by+the+Dozen%22",
"tweet_volume": null
},
{
"name": "Lovie",
"url": "http://twitter.com/search?q=Lovie",
"promoted_content": null,
"query": "Lovie",
"tweet_volume": 20737
},
{
"name": "Leslie Jones",
"url": "http://twitter.com/search?q=%22Leslie+Jones%22",
"promoted_content": null,
"query": "%22Leslie+Jones%22",
"tweet_volume": null
},
{
"name": "Lids",
"url": "http://twitter.com/search?q=Lids",
"promoted_content": null,
"query": "Lids",
"tweet_volume": null
},
{
"name": "#Bussin",
"url": "http://twitter.com/search?q=%23Bussin",
"promoted_content": null,
"query": "%23Bussin",
"tweet_volume": 14103
},
{
"name": "Sam Harris",
"url": "http://twitter.com/search?q=%22Sam+Harris%22",
"promoted_content": null,
"query": "%22Sam+Harris%22",
"tweet_volume": null
},
{
"name": "BTS PAVED THE WAY",
"url": "http://twitter.com/search?q=%22BTS+PAVED+THE+WAY%22",
"promoted_content": null,
"query": "%22BTS+PAVED+THE+WAY%22",
"tweet_volume": 15926
},
{
"name": "AIDS Awareness Day",
"url": "http://twitter.com/search?q=%22AIDS+Awareness+Day%22",
"promoted_content": null,
"query": "%22AIDS+Awareness+Day%22",
"tweet_volume": null
},
{
"name": "Ryan Clark",
"url": "http://twitter.com/search?q=%22Ryan+Clark%22",
"promoted_content": null,
"query": "%22Ryan+Clark%22",
"tweet_volume": null
},
{
"name": "Joe Rogan $100",
"url": "http://twitter.com/search?q=%22Joe+Rogan+%24100%22",
"promoted_content": null,
"query": "%22Joe+Rogan+%24100%22",
"tweet_volume": 20837
},
{
"name": "Jonah Goldberg",
"url": "http://twitter.com/search?q=%22Jonah+Goldberg%22",
"promoted_content": null,
"query": "%22Jonah+Goldberg%22",
"tweet_volume": null
},
{
"name": "AP Poll",
"url": "http://twitter.com/search?q=%22AP+Poll%22",
"promoted_content": null,
"query": "%22AP+Poll%22",
"tweet_volume": null
},
{
"name": "J Dilla",
"url": "http://twitter.com/search?q=%22J+Dilla%22",
"promoted_content": null,
"query": "%22J+Dilla%22",
"tweet_volume": null
},
{
"name": "Blazing Saddles",
"url": "http://twitter.com/search?q=%22Blazing+Saddles%22",
"promoted_content": null,
"query": "%22Blazing+Saddles%22",
"tweet_volume": null
},
{
"name": "Stitt",
"url": "http://twitter.com/search?q=Stitt",
"promoted_content": null,
"query": "Stitt",
"tweet_volume": null
},
{
"name": "Rumble",
"url": "http://twitter.com/search?q=Rumble",
"promoted_content": null,
"query": "Rumble",
"tweet_volume": 68251
},
{
"name": "Pulp Fiction",
"url": "http://twitter.com/search?q=%22Pulp+Fiction%22",
"promoted_content": null,
"query": "%22Pulp+Fiction%22",
"tweet_volume": null
},
{
"name": "Platinum",
"url": "http://twitter.com/search?q=Platinum",
"promoted_content": null,
"query": "Platinum",
"tweet_volume": 34523
},
{
"name": "ExtraordINary DAY",
"url": "http://twitter.com/search?q=%22ExtraordINary+DAY%22",
"promoted_content": null,
"query": "%22ExtraordINary+DAY%22",
"tweet_volume": 190470
},
{
"name": "KPMG",
"url": "http://twitter.com/search?q=KPMG",
"promoted_content": null,
"query": "KPMG",
"tweet_volume": 12431
},
{
"name": "Eric Andre",
"url": "http://twitter.com/search?q=%22Eric+Andre%22",
"promoted_content": null,
"query": "%22Eric+Andre%22",
"tweet_volume": null
},
{
"name": "Sharpe",
"url": "http://twitter.com/search?q=Sharpe",
"promoted_content": null,
"query": "Sharpe",
"tweet_volume": null
},
{
"name": "Odysee",
"url": "http://twitter.com/search?q=Odysee",
"promoted_content": null,
"query": "Odysee",
"tweet_volume": null
},
{
"name": "Deflategate",
"url": "http://twitter.com/search?q=Deflategate",
"promoted_content": null,
"query": "Deflategate",
"tweet_volume": null
},
{
"name": "Toney",
"url": "http://twitter.com/search?q=Toney",
"promoted_content": null,
"query": "Toney",
"tweet_volume": null
},
{
"name": "Chyna",
"url": "http://twitter.com/search?q=Chyna",
"promoted_content": null,
"query": "Chyna",
"tweet_volume": null
},
{
"name": "HAPPY BIRTHDAY JACK",
"url": "http://twitter.com/search?q=%22HAPPY+BIRTHDAY+JACK%22",
"promoted_content": null,
"query": "%22HAPPY+BIRTHDAY+JACK%22",
"tweet_volume": null
},
{
"name": "Eric Adams",
"url": "http://twitter.com/search?q=%22Eric+Adams%22",
"promoted_content": null,
"query": "%22Eric+Adams%22",
"tweet_volume": null
},
{
"name": "Player of the Week",
"url": "http://twitter.com/search?q=%22Player+of+the+Week%22",
"promoted_content": null,
"query": "%22Player+of+the+Week%22",
"tweet_volume": null
},
{
"name": "Essek",
"url": "http://twitter.com/search?q=Essek",
"promoted_content": null,
"query": "Essek",
"tweet_volume": null
},
{
"name": "Babylon's Fall",
"url": "http://twitter.com/search?q=%22Babylon%27s+Fall%22",
"promoted_content": null,
"query": "%22Babylon%27s+Fall%22",
"tweet_volume": null
},
{
"name": "Today is National Black HIV",
"url": "http://twitter.com/search?q=%22Today+is+National+Black+HIV%22",
"promoted_content": null,
"query": "%22Today+is+National+Black+HIV%22",
"tweet_volume": null
},
{
"name": "LOCK HIM UP",
"url": "http://twitter.com/search?q=%22LOCK+HIM+UP%22",
"promoted_content": null,
"query": "%22LOCK+HIM+UP%22",
"tweet_volume": null
},
{
"name": "Bruce Willis",
"url": "http://twitter.com/search?q=%22Bruce+Willis%22",
"promoted_content": null,
"query": "%22Bruce+Willis%22",
"tweet_volume": null
},
{
"name": "Vincent Zhou",
"url": "http://twitter.com/search?q=%22Vincent+Zhou%22",
"promoted_content": null,
"query": "%22Vincent+Zhou%22",
"tweet_volume": null
},
{
"name": "Bill Simmons",
"url": "http://twitter.com/search?q=%22Bill+Simmons%22",
"promoted_content": null,
"query": "%22Bill+Simmons%22",
"tweet_volume": null
},
{
"name": "DNCE",
"url": "http://twitter.com/search?q=DNCE",
"promoted_content": null,
"query": "DNCE",
"tweet_volume": null
}
],
"as_of": "2022-02-07T19:14:53Z",
"created_at": "2022-02-06T13:56:05Z",
"locations": [
{
"name": "United States",
"woeid": 23424977
}
]
}
]
Computing the intersection of two sets of trends#
world_trends_set = set([trend['name']
for trend in world_trends[0]['trends']])
us_trends_set = set([trend['name']
for trend in us_trends[0]['trends']])
common_trends = world_trends_set.intersection(us_trends_set)
print(common_trends)
{'Russell Brand', 'Cheaper by the Dozen', 'Pusha T', 'Frontier', 'KPMG', 'National Archives', 'Thibs', 'Lovie', '#MelodicVoiceINDay', 'Razzies', 'Tulsi Gabbard', 'ExtraordINary DAY', 'Sam Harris'}
Search Results#
# Set this variable to a trending topic,
# or anything else for that matter. The example query below
# was a trending topic when this content was being developed
# and is used throughout the remainder of this chapter.
import json
#q = "#Ada"
q = '#JasonRoy'
count = 1000
# See https://dev.twitter.com/docs/api/1.1/get/search/tweets
search_results = mathcs_twitter_api.search.tweets(q=q, count=count)
statuses = search_results['statuses']
# Iterate through 5 more batches of results by following the cursor
for _ in range(5):
print("Length of statuses", len(statuses))
try:
next_results = search_results['search_metadata']['next_results']
except KeyError: # No more results when next_results doesn't exist
break
# Create a dictionary from next_results, which has the following form:
# ?max_id=313519052523986943&q=NCAA&include_entities=1
kwargs = dict([ kv.split('=') for kv in next_results[1:].split("&") ])
search_results = mathcs_twitter_api.search.tweets(**kwargs)
statuses += search_results['statuses']
# Show one sample search result by slicing the list...
print(json.dumps(statuses, indent=4))
Streaming output truncated to the last 5000 lines.
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1186670941637611520,
"id_str": "1186670941637611520",
"name": "Cricket Room",
"screen_name": "cricketroom_",
"location": "Pakistan ",
"description": "official Twitter account - Cricket Room | https://t.co/VuDplRCcUS | https://t.co/Y76A6Cq53c",
"url": "https://t.co/Ez4BZJ78Am",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/Ez4BZJ78Am",
"expanded_url": "https://www.youtube.com/c/CricketRoompk?sub_confirmation=1",
"display_url": "youtube.com/c/CricketRoomp\u2026",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": [
{
"url": "https://t.co/VuDplRCcUS",
"expanded_url": "http://fb.com/cricketroompk",
"display_url": "fb.com/cricketroompk",
"indices": [
42,
65
]
},
{
"url": "https://t.co/Y76A6Cq53c",
"expanded_url": "http://instagram.com/cricketroom",
"display_url": "instagram.com/cricketroom",
"indices": [
68,
91
]
}
]
}
},
"protected": false,
"followers_count": 2795,
"friends_count": 19,
"listed_count": 3,
"created_at": "Tue Oct 22 15:50:54 +0000 2019",
"favourites_count": 3394,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 5764,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1484894392100040709/tuuwC21R_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1484894392100040709/tuuwC21R_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1186670941637611520/1643024264",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 3,
"favorite_count": 25,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
"is_quote_status": false,
"retweet_count": 3,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:39 +0000 2022",
"id": 1490755151187312643,
"id_str": "1490755151187312643",
"text": "Jason Roy received the Player Of The Match Award for his match-winning hundred.\n\n#JasonRoy #QuettaGladiators\u2026 https://t.co/ujDKye93V1",
"truncated": true,
"entities": {
"hashtags": [
{
"text": "JasonRoy",
"indices": [
81,
90
]
},
{
"text": "QuettaGladiators",
"indices": [
91,
108
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [
{
"url": "https://t.co/ujDKye93V1",
"expanded_url": "https://twitter.com/i/web/status/1490755151187312643",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
110,
133
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"https://mobile.twitter.com\" rel=\"nofollow\">Twitter Web App</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1213434794907815936,
"id_str": "1213434794907815936",
"name": "Bet Barter",
"screen_name": "BetBarteronline",
"location": "",
"description": "Follow us for all the latest happenings of sporting events across the globe.",
"url": "https://t.co/mtNLWTAzIF",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/mtNLWTAzIF",
"expanded_url": "https://betbarter.net/",
"display_url": "betbarter.net",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 1828,
"friends_count": 248,
"listed_count": 1,
"created_at": "Sat Jan 04 12:19:43 +0000 2020",
"favourites_count": 49,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 4892,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1227197996669923328/ZPjczzqB_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1227197996669923328/ZPjczzqB_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1213434794907815936/1643289714",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 1,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:33 +0000 2022",
"id": 1490755125425709062,
"id_str": "1490755125425709062",
"text": "Whattt a matchhh!!!\n\nWhat a victory @TeamQuetta\n@JasonRoy20 \ud83d\udcaf\n@lahoreqalandars better luck next tym\u2764\ufe0f\n\n#PSL7\u2026 https://t.co/rNzoDYCpk2",
"truncated": true,
"entities": {
"hashtags": [
{
"text": "PSL7",
"indices": [
103,
108
]
}
],
"symbols": [],
"user_mentions": [
{
"screen_name": "TeamQuetta",
"name": "Quetta Gladiators",
"id": 4405094896,
"id_str": "4405094896",
"indices": [
36,
47
]
},
{
"screen_name": "JasonRoy20",
"name": "Jason Roy",
"id": 343512172,
"id_str": "343512172",
"indices": [
48,
59
]
},
{
"screen_name": "lahoreqalandars",
"name": "Lahore Qalandars",
"id": 4402375702,
"id_str": "4402375702",
"indices": [
62,
78
]
}
],
"urls": [
{
"url": "https://t.co/rNzoDYCpk2",
"expanded_url": "https://twitter.com/i/web/status/1490755125425709062",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
110,
133
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1031163366969102336,
"id_str": "1031163366969102336",
"name": "Bushra Butt",
"screen_name": "BushraB30430471",
"location": "",
"description": "",
"url": "https://t.co/lo6k1LPhwk",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/lo6k1LPhwk",
"expanded_url": "https://www.instagram.com/p/CVYOKHys70s/?utm_medium=copy_link",
"display_url": "instagram.com/p/CVYOKHys70s/\u2026",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 5,
"friends_count": 49,
"listed_count": 0,
"created_at": "Sun Aug 19 12:57:59 +0000 2018",
"favourites_count": 59,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 14,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1475158326811967495/EY1q6Zd5_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1475158326811967495/EY1q6Zd5_normal.jpg",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:32 +0000 2022",
"id": 1490755120501440516,
"id_str": "1490755120501440516",
"text": "Beast Form @JasonRoy20 \ud83e\udd29\ud83d\udd25\ud83d\udca5\ud83d\udd7a\n\n#JasonRoy #PSL7 https://t.co/4aNDXLviQd",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "JasonRoy",
"indices": [
29,
38
]
},
{
"text": "PSL7",
"indices": [
39,
44
]
}
],
"symbols": [],
"user_mentions": [
{
"screen_name": "JasonRoy20",
"name": "Jason Roy",
"id": 343512172,
"id_str": "343512172",
"indices": [
11,
22
]
}
],
"urls": [],
"media": [
{
"id": 1490755108371841025,
"id_str": "1490755108371841025",
"indices": [
45,
68
],
"media_url": "http://pbs.twimg.com/media/FLA532maQAE_swq.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA532maQAE_swq.jpg",
"url": "https://t.co/4aNDXLviQd",
"display_url": "pic.twitter.com/4aNDXLviQd",
"expanded_url": "https://twitter.com/barathraina567/status/1490755120501440516/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 676,
"resize": "fit"
},
"large": {
"w": 1913,
"h": 1078,
"resize": "fit"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490755108371841025,
"id_str": "1490755108371841025",
"indices": [
45,
68
],
"media_url": "http://pbs.twimg.com/media/FLA532maQAE_swq.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA532maQAE_swq.jpg",
"url": "https://t.co/4aNDXLviQd",
"display_url": "pic.twitter.com/4aNDXLviQd",
"expanded_url": "https://twitter.com/barathraina567/status/1490755120501440516/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 676,
"resize": "fit"
},
"large": {
"w": 1913,
"h": 1078,
"resize": "fit"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1374715891766554625,
"id_str": "1374715891766554625",
"name": "MASTER\ud83d\ude0e",
"screen_name": "barathraina567",
"location": "Pondicherry, India",
"description": "Ardent Fan Of @actorvijay @Imraina Fan of @RishabhPant17 @SDsridivya @JasonRoy20 @Msdhoni #ARR #Vikram #GVP @Selvaraghavan Back Up @barathraina56 Suspended..",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 803,
"friends_count": 1040,
"listed_count": 0,
"created_at": "Wed Mar 24 13:33:13 +0000 2021",
"favourites_count": 14296,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 14417,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1490602985734684675/1fzz8df8_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1490602985734684675/1fzz8df8_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1374715891766554625/1642952488",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:31 +0000 2022",
"id": 1490755117683187712,
"id_str": "1490755117683187712",
"text": "RT @FatimaMasroor56: What a win Quetta! Magnificent Performance by Jason Roy. 116 on 57. Such a Classical Batsman. Perfect win over Qalanda\u2026",
"truncated": false,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [
{
"screen_name": "FatimaMasroor56",
"name": "Fatima Masroor",
"id": 1010534269784076290,
"id_str": "1010534269784076290",
"indices": [
3,
19
]
}
],
"urls": []
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 905381407576526848,
"id_str": "905381407576526848",
"name": "Amit",
"screen_name": "Amity4116",
"location": "Pakistan",
"description": "Obsessed with Cricket and Cats. Babar Azam and KL Rahul fan. Writing Odd Stuff. Introvert yet online extrovert. Feminist.",
"url": "https://t.co/9FX2ZfMQ9C",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/9FX2ZfMQ9C",
"expanded_url": "https://instagram.com/amity_writes?utm_medium=copy_link",
"display_url": "instagram.com/amity_writes?u\u2026",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 441,
"friends_count": 2367,
"listed_count": 0,
"created_at": "Wed Sep 06 10:45:23 +0000 2017",
"favourites_count": 16135,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 2223,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1489863960040022016/4aHJIShs_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1489863960040022016/4aHJIShs_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/905381407576526848/1639075703",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"retweeted_status": {
"created_at": "Mon Feb 07 18:30:59 +0000 2022",
"id": 1490754984861917190,
"id_str": "1490754984861917190",
"text": "What a win Quetta! Magnificent Performance by Jason Roy. 116 on 57. Such a Classical Batsman. Perfect win over Qala\u2026 https://t.co/NsSHveXYmq",
"truncated": true,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [],
"urls": [
{
"url": "https://t.co/NsSHveXYmq",
"expanded_url": "https://twitter.com/i/web/status/1490754984861917190",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
117,
140
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1010534269784076290,
"id_str": "1010534269784076290",
"name": "Fatima Masroor",
"screen_name": "FatimaMasroor56",
"location": "Rawalpindi, Pakistan",
"description": "18| Literature\ud83e\ude84| Not expert in Cricket but I know the game little bit| here for appreciating cover drives| THEY CANNOT PLAY HIM #LQ\ud83e\udd1e\ud83c\uddf5\ud83c\uddf0",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 4500,
"friends_count": 322,
"listed_count": 3,
"created_at": "Sat Jun 23 14:45:19 +0000 2018",
"favourites_count": 47015,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 41818,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1489946056024596481/8o7pi5QI_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1489946056024596481/8o7pi5QI_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1010534269784076290/1644227375",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 1,
"favorite_count": 13,
"favorited": false,
"retweeted": false,
"lang": "en"
},
"is_quote_status": false,
"retweet_count": 1,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:30 +0000 2022",
"id": 1490755113799008263,
"id_str": "1490755113799008263",
"text": "Quetta Gladiators's won by 7 wickets\ud83e\udd29 and this man has stolen the match\ud83d\udd25\nWeldone #PurpleForce \n#JasonRoy \n#QGvsLQ https://t.co/dXNudOIeIV",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "PurpleForce",
"indices": [
81,
93
]
},
{
"text": "JasonRoy",
"indices": [
95,
104
]
},
{
"text": "QGvsLQ",
"indices": [
106,
113
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490755111043444739,
"id_str": "1490755111043444739",
"indices": [
114,
137
],
"media_url": "http://pbs.twimg.com/media/FLA54AjXsAMp7Qr.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA54AjXsAMp7Qr.jpg",
"url": "https://t.co/dXNudOIeIV",
"display_url": "pic.twitter.com/dXNudOIeIV",
"expanded_url": "https://twitter.com/thatdiljali/status/1490755113799008263/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 676,
"resize": "fit"
},
"large": {
"w": 1913,
"h": 1078,
"resize": "fit"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490755111043444739,
"id_str": "1490755111043444739",
"indices": [
114,
137
],
"media_url": "http://pbs.twimg.com/media/FLA54AjXsAMp7Qr.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA54AjXsAMp7Qr.jpg",
"url": "https://t.co/dXNudOIeIV",
"display_url": "pic.twitter.com/dXNudOIeIV",
"expanded_url": "https://twitter.com/thatdiljali/status/1490755113799008263/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 676,
"resize": "fit"
},
"large": {
"w": 1913,
"h": 1078,
"resize": "fit"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1484180034147389441,
"id_str": "1484180034147389441",
"name": "Sawera",
"screen_name": "thatdiljali",
"location": "Balochistan ",
"description": "Just going against the race!",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 0,
"friends_count": 12,
"listed_count": 0,
"created_at": "Thu Jan 20 15:06:16 +0000 2022",
"favourites_count": 0,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 27,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1484187227802374147/Is7X0EN1_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1484187227802374147/Is7X0EN1_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1484180034147389441/1642692762",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 1,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:28 +0000 2022",
"id": 1490755104735121408,
"id_str": "1490755104735121408",
"text": "Lahore qalander fans RN \ud83e\udd23\ud83e\udd23\n https://t.co/mDKf2ea6fU\n#JasonRoy\n#QGvsLQ",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "JasonRoy",
"indices": [
52,
61
]
},
{
"text": "QGvsLQ",
"indices": [
62,
69
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1104437523244290048,
"id_str": "1104437523244290048",
"indices": [
28,
51
],
"media_url": "http://pbs.twimg.com/ext_tw_video_thumb/1104437523244290048/pu/img/1c0YIzhg1pIzOD35.jpg",
"media_url_https": "https://pbs.twimg.com/ext_tw_video_thumb/1104437523244290048/pu/img/1c0YIzhg1pIzOD35.jpg",
"url": "https://t.co/mDKf2ea6fU",
"display_url": "pic.twitter.com/mDKf2ea6fU",
"expanded_url": "https://twitter.com/PSLMemesWalay/status/1104437577061412868/video/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 675,
"resize": "fit"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
},
"large": {
"w": 1280,
"h": 720,
"resize": "fit"
}
},
"source_status_id": 1104437577061412868,
"source_status_id_str": "1104437577061412868",
"source_user_id": 4566046276,
"source_user_id_str": "4566046276"
}
]
},
"extended_entities": {
"media": [
{
"id": 1104437523244290048,
"id_str": "1104437523244290048",
"indices": [
28,
51
],
"media_url": "http://pbs.twimg.com/ext_tw_video_thumb/1104437523244290048/pu/img/1c0YIzhg1pIzOD35.jpg",
"media_url_https": "https://pbs.twimg.com/ext_tw_video_thumb/1104437523244290048/pu/img/1c0YIzhg1pIzOD35.jpg",
"url": "https://t.co/mDKf2ea6fU",
"display_url": "pic.twitter.com/mDKf2ea6fU",
"expanded_url": "https://twitter.com/PSLMemesWalay/status/1104437577061412868/video/1",
"type": "video",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 675,
"resize": "fit"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
},
"large": {
"w": 1280,
"h": 720,
"resize": "fit"
}
},
"source_status_id": 1104437577061412868,
"source_status_id_str": "1104437577061412868",
"source_user_id": 4566046276,
"source_user_id_str": "4566046276",
"video_info": {
"aspect_ratio": [
16,
9
],
"duration_millis": 7634,
"variants": [
{
"content_type": "application/x-mpegURL",
"url": "https://video.twimg.com/ext_tw_video/1104437523244290048/pu/pl/1Ikx3Z_1thMD0uQ4.m3u8?tag=8"
},
{
"bitrate": 256000,
"content_type": "video/mp4",
"url": "https://video.twimg.com/ext_tw_video/1104437523244290048/pu/vid/320x180/81Q1Pl3Mi6qNaw7Y.mp4?tag=8"
},
{
"bitrate": 2176000,
"content_type": "video/mp4",
"url": "https://video.twimg.com/ext_tw_video/1104437523244290048/pu/vid/1280x720/ADFYl3V1RdlLEJfZ.mp4?tag=8"
},
{
"bitrate": 832000,
"content_type": "video/mp4",
"url": "https://video.twimg.com/ext_tw_video/1104437523244290048/pu/vid/640x360/4qIeqHT7WHOzTvZH.mp4?tag=8"
}
]
},
"additional_media_info": {
"monetizable": false
}
}
]
},
"metadata": {
"iso_language_code": "da",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 450775756,
"id_str": "450775756",
"name": "Dead",
"screen_name": "its_shoaib1",
"location": "In ur Eyes ",
"description": "Dead",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 405,
"friends_count": 28,
"listed_count": 4,
"created_at": "Fri Dec 30 17:00:35 +0000 2011",
"favourites_count": 27043,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 11043,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "EBEBEB",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme7/bg.gif",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme7/bg.gif",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1079294002623639552/xgh46F7h_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1079294002623639552/xgh46F7h_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/450775756/1469177811",
"profile_link_color": "990000",
"profile_sidebar_border_color": "DFDFDF",
"profile_sidebar_fill_color": "F3F3F3",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": false,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 1,
"favorite_count": 2,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "da"
},
{
"created_at": "Mon Feb 07 18:31:25 +0000 2022",
"id": 1490755092148072451,
"id_str": "1490755092148072451",
"text": "RT @salaar_gul: \"Only big boys play at night.\"\n(Sir Vivian Richard)\nAnother nail biting finsh, what a great tournament #PSL7\n#QGvsLQ\n#Jason\u2026",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "PSL7",
"indices": [
119,
124
]
},
{
"text": "QGvsLQ",
"indices": [
125,
132
]
}
],
"symbols": [],
"user_mentions": [
{
"screen_name": "salaar_gul",
"name": "Qasim",
"id": 3268264236,
"id_str": "3268264236",
"indices": [
3,
14
]
}
],
"urls": []
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1462497325679583234,
"id_str": "1462497325679583234",
"name": "Hawk",
"screen_name": "Pak_Hawk47",
"location": "Pakistan",
"description": "Waiting for an app that could deliver six-packs \ud83d\udcaa",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 2,
"friends_count": 59,
"listed_count": 0,
"created_at": "Sun Nov 21 19:06:13 +0000 2021",
"favourites_count": 253,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 127,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1462497765825601539/TZB4mU97_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1462497765825601539/TZB4mU97_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1462497325679583234/1637526828",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"retweeted_status": {
"created_at": "Mon Feb 07 18:30:43 +0000 2022",
"id": 1490754914305421313,
"id_str": "1490754914305421313",
"text": "\"Only big boys play at night.\"\n(Sir Vivian Richard)\nAnother nail biting finsh, what a great tournament #PSL7\u2026 https://t.co/k63XCsyIU9",
"truncated": true,
"entities": {
"hashtags": [
{
"text": "PSL7",
"indices": [
103,
108
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [
{
"url": "https://t.co/k63XCsyIU9",
"expanded_url": "https://twitter.com/i/web/status/1490754914305421313",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
110,
133
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 3268264236,
"id_str": "3268264236",
"name": "Qasim",
"screen_name": "salaar_gul",
"location": "\u0627\u0631\u0636\u0650 \u067e\u0627\u06a9",
"description": "\u0627\u0650\u06cc\u064e\u0651\u0627\u06a9\u064e \u0646\u064e\u0639\u06e1\u0628\u064f\u062f\u064f \u0648\u064e \u0627\u0650\u06cc\u064e\u0651\u0627\u06a9\u064e \u0646\u064e\u0633\u06e1\u062a\u064e\u0639\u0650\u06cc\u06e1\u0646\u064f \u0615",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 1003,
"friends_count": 1653,
"listed_count": 1,
"created_at": "Sat Jul 04 17:40:49 +0000 2015",
"favourites_count": 16473,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 4463,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1488104732824686594/k-tvNJ4f_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1488104732824686594/k-tvNJ4f_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/3268264236/1641410138",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 2,
"favorite_count": 4,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
"is_quote_status": false,
"retweet_count": 2,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:17 +0000 2022",
"id": 1490755059688300548,
"id_str": "1490755059688300548",
"text": "What a Amazing \u2665\ufe0f well Played Jason Roy. you Are Beuty,\ud83c\udfcf\u2665\ufe0f\ud83d\udc4f\n#QGvsLQ \n#JasonRoy https://t.co/8QHYaMIQT3",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "QGvsLQ",
"indices": [
60,
67
]
},
{
"text": "JasonRoy",
"indices": [
69,
78
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490755054550323202,
"id_str": "1490755054550323202",
"indices": [
79,
102
],
"media_url": "http://pbs.twimg.com/media/FLA50uGXEAIkrO2.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA50uGXEAIkrO2.jpg",
"url": "https://t.co/8QHYaMIQT3",
"display_url": "pic.twitter.com/8QHYaMIQT3",
"expanded_url": "https://twitter.com/PrinceZaheerAw2/status/1490755059688300548/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 452,
"h": 678,
"resize": "fit"
},
"small": {
"w": 452,
"h": 678,
"resize": "fit"
},
"large": {
"w": 452,
"h": 678,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490755054550323202,
"id_str": "1490755054550323202",
"indices": [
79,
102
],
"media_url": "http://pbs.twimg.com/media/FLA50uGXEAIkrO2.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA50uGXEAIkrO2.jpg",
"url": "https://t.co/8QHYaMIQT3",
"display_url": "pic.twitter.com/8QHYaMIQT3",
"expanded_url": "https://twitter.com/PrinceZaheerAw2/status/1490755059688300548/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 452,
"h": 678,
"resize": "fit"
},
"small": {
"w": 452,
"h": 678,
"resize": "fit"
},
"large": {
"w": 452,
"h": 678,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 782487616465711104,
"id_str": "782487616465711104",
"name": "@Zaheer Awan",
"screen_name": "PrinceZaheerAw2",
"location": "Karachi Pakistan",
"description": "Proud be a Pakistani\n\n*_\u0646\u06c1 \u0639\u0631\u0648\u062c \u0627\u0686\u06be\u0627 \u0646\u06c1 \u0632\u0648\u0627\u0644 \u0627\u0686\u06be\u0627 _*\ud83c\udfb6\n*_\u062c\u0633 \u062d\u0627\u0644 \u0645\u06cc\u06ba \u062e\u062f\u0627 \u0631\u06a9\u06be\u06d2 \u0648\u06c1\u06cc \u062d\u0627\u0644 \u0627\u0686\u06be\u0627 _*\u2764\ufe0f",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 10,
"friends_count": 13,
"listed_count": 0,
"created_at": "Sun Oct 02 07:49:20 +0000 2016",
"favourites_count": 156,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 74,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1481284141333291014/wsYFTie1_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1481284141333291014/wsYFTie1_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/782487616465711104/1596900552",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 1,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:07 +0000 2022",
"id": 1490755016575102980,
"id_str": "1490755016575102980",
"text": "What a well knitted performance #QuettaGladiators well done #JasonRoy @ivivianrichards \"Only big boys play at nigh\u2026 https://t.co/CKPa221ZS3",
"truncated": true,
"entities": {
"hashtags": [
{
"text": "QuettaGladiators",
"indices": [
32,
49
]
},
{
"text": "JasonRoy",
"indices": [
60,
69
]
}
],
"symbols": [],
"user_mentions": [
{
"screen_name": "ivivianrichards",
"name": "Sir Vivian Richards",
"id": 833618012162895873,
"id_str": "833618012162895873",
"indices": [
71,
87
]
}
],
"urls": [
{
"url": "https://t.co/CKPa221ZS3",
"expanded_url": "https://twitter.com/i/web/status/1490755016575102980",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
117,
140
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 292997899,
"id_str": "292997899",
"name": "shazia saqib habib",
"screen_name": "Shaz004",
"location": "",
"description": "Owner & Editor @fuchsia_mag follow if you like what I say, and even if you don't!",
"url": "https://t.co/VlQqUQQ3Mh",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/VlQqUQQ3Mh",
"expanded_url": "http://www.fuchsiamagazine.com",
"display_url": "fuchsiamagazine.com",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 273,
"friends_count": 187,
"listed_count": 4,
"created_at": "Wed May 04 15:53:35 +0000 2011",
"favourites_count": 1999,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 1344,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1449398519681495047/hsnZ6cDP_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1449398519681495047/hsnZ6cDP_normal.jpg",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:04 +0000 2022",
"id": 1490755003719376896,
"id_str": "1490755003719376896",
"text": "Fastest 100's of psl \n#PSL7 #JasonRoy https://t.co/8Y1Fc1voHQ",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "PSL7",
"indices": [
22,
27
]
},
{
"text": "JasonRoy",
"indices": [
28,
37
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490754999386841090,
"id_str": "1490754999386841090",
"indices": [
38,
61
],
"media_url": "http://pbs.twimg.com/media/FLA5xgmXIAIC_Dv.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5xgmXIAIC_Dv.jpg",
"url": "https://t.co/8Y1Fc1voHQ",
"display_url": "pic.twitter.com/8Y1Fc1voHQ",
"expanded_url": "https://twitter.com/alizulqarnainb3/status/1490755003719376896/photo/1",
"type": "photo",
"sizes": {
"medium": {
"w": 720,
"h": 405,
"resize": "fit"
},
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
},
"large": {
"w": 720,
"h": 405,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754999386841090,
"id_str": "1490754999386841090",
"indices": [
38,
61
],
"media_url": "http://pbs.twimg.com/media/FLA5xgmXIAIC_Dv.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5xgmXIAIC_Dv.jpg",
"url": "https://t.co/8Y1Fc1voHQ",
"display_url": "pic.twitter.com/8Y1Fc1voHQ",
"expanded_url": "https://twitter.com/alizulqarnainb3/status/1490755003719376896/photo/1",
"type": "photo",
"sizes": {
"medium": {
"w": 720,
"h": 405,
"resize": "fit"
},
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
},
"large": {
"w": 720,
"h": 405,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 825399399778021376,
"id_str": "825399399778021376",
"name": "Ali ZulqarnainBhatti",
"screen_name": "alizulqarnainb3",
"location": "Lahore, Pakistan",
"description": "",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 136,
"friends_count": 558,
"listed_count": 0,
"created_at": "Sat Jan 28 17:45:26 +0000 2017",
"favourites_count": 10834,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 1030,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1377213922479116289/5NvnIE5M_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1377213922479116289/5NvnIE5M_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/825399399778021376/1599975142",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 1,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:31:00 +0000 2022",
"id": 1490754987382788098,
"id_str": "1490754987382788098",
"text": "RT @CricksLab: 'He Came, He Saw, He Conquered' \n\n@JasonRoy20 giving high hopes to the Quetta Gladiators as they beat Lahore Qalandars by 7\u2026",
"truncated": false,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [
{
"screen_name": "CricksLab",
"name": "CricksLab",
"id": 950368378182688768,
"id_str": "950368378182688768",
"indices": [
3,
13
]
},
{
"screen_name": "JasonRoy20",
"name": "Jason Roy",
"id": 343512172,
"id_str": "343512172",
"indices": [
49,
60
]
}
],
"urls": []
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 3293730065,
"id_str": "3293730065",
"name": "Asif\ud83d\udc26QG\ud83d\udc9c",
"screen_name": "OyeSiffy",
"location": "Between here &there",
"description": "\u200f\u200f\u200f\u200f\u200f\u200f\u200f\u200f\u200f\u200f\u200f\u200f\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e#NFAK \u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e#Cricket\u2764 \u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u0628\u0644\u0648\u0686\n\u200e\u200e\u200e\u200e\u200e\u200e\u200e\u200e#Iubian.Ryk\ud83c\udf93",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 900,
"friends_count": 504,
"listed_count": 1,
"created_at": "Fri May 22 06:27:18 +0000 2015",
"favourites_count": 260640,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 93305,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1488760909484941313/4Zxh2Ngp_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1488760909484941313/4Zxh2Ngp_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/3293730065/1533621987",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"retweeted_status": {
"created_at": "Mon Feb 07 18:26:46 +0000 2022",
"id": 1490753922767618049,
"id_str": "1490753922767618049",
"text": "'He Came, He Saw, He Conquered' \n\n@JasonRoy20 giving high hopes to the Quetta Gladiators as they beat Lahore Qaland\u2026 https://t.co/ZFbKmo3EmZ",
"truncated": true,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [
{
"screen_name": "JasonRoy20",
"name": "Jason Roy",
"id": 343512172,
"id_str": "343512172",
"indices": [
34,
45
]
}
],
"urls": [
{
"url": "https://t.co/ZFbKmo3EmZ",
"expanded_url": "https://twitter.com/i/web/status/1490753922767618049",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
117,
140
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 950368378182688768,
"id_str": "950368378182688768",
"name": "CricksLab",
"screen_name": "CricksLab",
"location": "Dubai, UAE",
"description": "The World's # 1 Cricket Management Platform | Live Scores | HD Graphics | Live Streaming | Player Auction Module | Team Profiles | Advance Insights | Stats |",
"url": "https://t.co/GG05sAFs48",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/GG05sAFs48",
"expanded_url": "https://crickslab.com",
"display_url": "crickslab.com",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 1316,
"friends_count": 28,
"listed_count": 2,
"created_at": "Mon Jan 08 14:07:33 +0000 2018",
"favourites_count": 32,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 446,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "000000",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1486659742965673985/zHQ-RMBm_normal.png",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1486659742965673985/zHQ-RMBm_normal.png",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/950368378182688768/1643282297",
"profile_link_color": "1B95E0",
"profile_sidebar_border_color": "000000",
"profile_sidebar_fill_color": "000000",
"profile_text_color": "000000",
"profile_use_background_image": false,
"has_extended_profile": false,
"default_profile": false,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 7,
"favorite_count": 68,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
"is_quote_status": false,
"retweet_count": 7,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:59 +0000 2022",
"id": 1490754984861917190,
"id_str": "1490754984861917190",
"text": "What a win Quetta! Magnificent Performance by Jason Roy. 116 on 57. Such a Classical Batsman. Perfect win over Qala\u2026 https://t.co/NsSHveXYmq",
"truncated": true,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [],
"urls": [
{
"url": "https://t.co/NsSHveXYmq",
"expanded_url": "https://twitter.com/i/web/status/1490754984861917190",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
117,
140
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1010534269784076290,
"id_str": "1010534269784076290",
"name": "Fatima Masroor",
"screen_name": "FatimaMasroor56",
"location": "Rawalpindi, Pakistan",
"description": "18| Literature\ud83e\ude84| Not expert in Cricket but I know the game little bit| here for appreciating cover drives| THEY CANNOT PLAY HIM #LQ\ud83e\udd1e\ud83c\uddf5\ud83c\uddf0",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 4500,
"friends_count": 322,
"listed_count": 3,
"created_at": "Sat Jun 23 14:45:19 +0000 2018",
"favourites_count": 47015,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 41818,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1489946056024596481/8o7pi5QI_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1489946056024596481/8o7pi5QI_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1010534269784076290/1644227375",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 1,
"favorite_count": 13,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:58 +0000 2022",
"id": 1490754980449599492,
"id_str": "1490754980449599492",
"text": "What a blasting Entry of #JasonRoy in #QuettaGladiators in #PSL7.\n116 runs in just 57 ball.\nWhat a class. https://t.co/eTlAG074Dl",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "JasonRoy",
"indices": [
25,
34
]
},
{
"text": "QuettaGladiators",
"indices": [
38,
55
]
},
{
"text": "PSL7",
"indices": [
59,
64
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490754976871817221,
"id_str": "1490754976871817221",
"indices": [
106,
129
],
"media_url": "http://pbs.twimg.com/media/FLA5wMuXIAUfznQ.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5wMuXIAUfznQ.jpg",
"url": "https://t.co/eTlAG074Dl",
"display_url": "pic.twitter.com/eTlAG074Dl",
"expanded_url": "https://twitter.com/SaqibRa08506448/status/1490754980449599492/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"large": {
"w": 452,
"h": 254,
"resize": "fit"
},
"small": {
"w": 452,
"h": 254,
"resize": "fit"
},
"medium": {
"w": 452,
"h": 254,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754976871817221,
"id_str": "1490754976871817221",
"indices": [
106,
129
],
"media_url": "http://pbs.twimg.com/media/FLA5wMuXIAUfznQ.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5wMuXIAUfznQ.jpg",
"url": "https://t.co/eTlAG074Dl",
"display_url": "pic.twitter.com/eTlAG074Dl",
"expanded_url": "https://twitter.com/SaqibRa08506448/status/1490754980449599492/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"large": {
"w": 452,
"h": 254,
"resize": "fit"
},
"small": {
"w": 452,
"h": 254,
"resize": "fit"
},
"medium": {
"w": 452,
"h": 254,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1427889834748743688,
"id_str": "1427889834748743688",
"name": "Saqib Rajput",
"screen_name": "SaqibRa08506448",
"location": "",
"description": "No Religion is higher than Humanity...!!!",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 163,
"friends_count": 1052,
"listed_count": 0,
"created_at": "Wed Aug 18 07:07:53 +0000 2021",
"favourites_count": 31510,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 3953,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1485145078062628865/fTxyihMD_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1485145078062628865/fTxyihMD_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1427889834748743688/1641801364",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 2,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:55 +0000 2022",
"id": 1490754964238573583,
"id_str": "1490754964238573583",
"text": "@thePSLt20 Lahore Qalandar Fans Watching Jason Roy\u2019s Batting\ud83d\ude02\n#jasonroy \n#PSLAnthem \n#QGvMS https://t.co/ivXDHK8gXW",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "jasonroy",
"indices": [
62,
71
]
},
{
"text": "PSLAnthem",
"indices": [
73,
83
]
},
{
"text": "QGvMS",
"indices": [
85,
91
]
}
],
"symbols": [],
"user_mentions": [
{
"screen_name": "thePSLt20",
"name": "PakistanSuperLeague",
"id": 3448716797,
"id_str": "3448716797",
"indices": [
0,
10
]
}
],
"urls": [],
"media": [
{
"id": 1490754961369714688,
"id_str": "1490754961369714688",
"indices": [
92,
115
],
"media_url": "http://pbs.twimg.com/media/FLA5vS-X0AAVgjO.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5vS-X0AAVgjO.jpg",
"url": "https://t.co/ivXDHK8gXW",
"display_url": "pic.twitter.com/ivXDHK8gXW",
"expanded_url": "https://twitter.com/fayazhussain177/status/1490754964238573583/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 720,
"h": 405,
"resize": "fit"
},
"large": {
"w": 720,
"h": 405,
"resize": "fit"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754961369714688,
"id_str": "1490754961369714688",
"indices": [
92,
115
],
"media_url": "http://pbs.twimg.com/media/FLA5vS-X0AAVgjO.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5vS-X0AAVgjO.jpg",
"url": "https://t.co/ivXDHK8gXW",
"display_url": "pic.twitter.com/ivXDHK8gXW",
"expanded_url": "https://twitter.com/fayazhussain177/status/1490754964238573583/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 720,
"h": 405,
"resize": "fit"
},
"large": {
"w": 720,
"h": 405,
"resize": "fit"
},
"small": {
"w": 680,
"h": 383,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": 1490754219187527682,
"in_reply_to_status_id_str": "1490754219187527682",
"in_reply_to_user_id": 3448716797,
"in_reply_to_user_id_str": "3448716797",
"in_reply_to_screen_name": "thePSLt20",
"user": {
"id": 813975371033014272,
"id_str": "813975371033014272",
"name": "Fayaz Hussain",
"screen_name": "fayazhussain177",
"location": "uthal",
"description": "studying",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 129,
"friends_count": 453,
"listed_count": 0,
"created_at": "Wed Dec 28 05:10:26 +0000 2016",
"favourites_count": 1331,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 510,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1455232096281186305/TL77tVAn_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1455232096281186305/TL77tVAn_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/813975371033014272/1643825305",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 2,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:54 +0000 2022",
"id": 1490754961042546693,
"id_str": "1490754961042546693",
"text": "HAPPY TAPTAAN \ud83d\ude06\ud83d\ude01\ud83d\ude02\ud83e\udd23\n\n#GQvsLQ #Lahore #jasonroy https://t.co/zW087gdmeU",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "GQvsLQ",
"indices": [
20,
27
]
},
{
"text": "Lahore",
"indices": [
28,
35
]
},
{
"text": "jasonroy",
"indices": [
36,
45
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490754957154398209,
"id_str": "1490754957154398209",
"indices": [
46,
69
],
"media_url": "http://pbs.twimg.com/media/FLA5vDRXMAESKpH.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5vDRXMAESKpH.jpg",
"url": "https://t.co/zW087gdmeU",
"display_url": "pic.twitter.com/zW087gdmeU",
"expanded_url": "https://twitter.com/abbasshere/status/1490754961042546693/photo/1",
"type": "photo",
"sizes": {
"large": {
"w": 1024,
"h": 869,
"resize": "fit"
},
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1024,
"h": 869,
"resize": "fit"
},
"small": {
"w": 680,
"h": 577,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754957154398209,
"id_str": "1490754957154398209",
"indices": [
46,
69
],
"media_url": "http://pbs.twimg.com/media/FLA5vDRXMAESKpH.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5vDRXMAESKpH.jpg",
"url": "https://t.co/zW087gdmeU",
"display_url": "pic.twitter.com/zW087gdmeU",
"expanded_url": "https://twitter.com/abbasshere/status/1490754961042546693/photo/1",
"type": "photo",
"sizes": {
"large": {
"w": 1024,
"h": 869,
"resize": "fit"
},
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1024,
"h": 869,
"resize": "fit"
},
"small": {
"w": 680,
"h": 577,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "nl",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 722096382,
"id_str": "722096382",
"name": "\ud835\ude3c\ud835\ude3d\ud835\ude3d\ud835\ude3c\ud835\ude4e \ud83d\udc51",
"screen_name": "abbasshere",
"location": "KARBALA",
"description": "\u064a\u0627 \u0627\u0628\u0627 \u0627\u0644\u0641\u0636\u0644 \u0627\u0644\u0639\u0628\u0627\u0633\ufdfb",
"url": "https://t.co/m49Un36MuA",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/m49Un36MuA",
"expanded_url": "http://facebook.com/iamabbass",
"display_url": "facebook.com/iamabbass",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 306,
"friends_count": 218,
"listed_count": 0,
"created_at": "Sat Jul 28 12:33:45 +0000 2012",
"favourites_count": 2496,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 1199,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "000000",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1488839428810416128/4iprDsgc_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1488839428810416128/4iprDsgc_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/722096382/1642147416",
"profile_link_color": "981CEB",
"profile_sidebar_border_color": "000000",
"profile_sidebar_fill_color": "000000",
"profile_text_color": "000000",
"profile_use_background_image": false,
"has_extended_profile": true,
"default_profile": false,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 1,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "nl"
},
{
"created_at": "Mon Feb 07 18:30:48 +0000 2022",
"id": 1490754938229739524,
"id_str": "1490754938229739524",
"text": "What an intro by #JASONROY.\nGreat match b/w #QGvsLQ\nAfter a long time we have seen the spark of QG.",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "JASONROY",
"indices": [
17,
26
]
},
{
"text": "QGvsLQ",
"indices": [
44,
51
]
}
],
"symbols": [],
"user_mentions": [],
"urls": []
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1470061497397043207,
"id_str": "1470061497397043207",
"name": "_experto_",
"screen_name": "experto971",
"location": "",
"description": "Swimmer,",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 0,
"friends_count": 14,
"listed_count": 0,
"created_at": "Sun Dec 12 16:02:41 +0000 2021",
"favourites_count": 4,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 15,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1470061805921652737/fW8gEKR7_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1470061805921652737/fW8gEKR7_normal.jpg",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:43 +0000 2022",
"id": 1490754914305421313,
"id_str": "1490754914305421313",
"text": "\"Only big boys play at night.\"\n(Sir Vivian Richard)\nAnother nail biting finsh, what a great tournament #PSL7\u2026 https://t.co/k63XCsyIU9",
"truncated": true,
"entities": {
"hashtags": [
{
"text": "PSL7",
"indices": [
103,
108
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [
{
"url": "https://t.co/k63XCsyIU9",
"expanded_url": "https://twitter.com/i/web/status/1490754914305421313",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
110,
133
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 3268264236,
"id_str": "3268264236",
"name": "Qasim",
"screen_name": "salaar_gul",
"location": "\u0627\u0631\u0636\u0650 \u067e\u0627\u06a9",
"description": "\u0627\u0650\u06cc\u064e\u0651\u0627\u06a9\u064e \u0646\u064e\u0639\u06e1\u0628\u064f\u062f\u064f \u0648\u064e \u0627\u0650\u06cc\u064e\u0651\u0627\u06a9\u064e \u0646\u064e\u0633\u06e1\u062a\u064e\u0639\u0650\u06cc\u06e1\u0646\u064f \u0615",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 1003,
"friends_count": 1653,
"listed_count": 1,
"created_at": "Sat Jul 04 17:40:49 +0000 2015",
"favourites_count": 16473,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 4463,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1488104732824686594/k-tvNJ4f_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1488104732824686594/k-tvNJ4f_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/3268264236/1641410138",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 2,
"favorite_count": 4,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:36 +0000 2022",
"id": 1490754885532491781,
"id_str": "1490754885532491781",
"text": "RT @Ayemanmalik01: Roy oh My Boyyy\ud83d\udd25\ud83e\udd2f\ud83e\udd29What an Innings BrOooooo\ud83e\udd18\ud83d\ude4c\n\n#LQvQG #QGvLQ #Cricket #PSL2022 #SarfarazAhmed #jasonroy https://t.co/dGvi\u2026",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "LQvQG",
"indices": [
65,
71
]
},
{
"text": "QGvLQ",
"indices": [
72,
78
]
},
{
"text": "Cricket",
"indices": [
79,
87
]
},
{
"text": "PSL2022",
"indices": [
88,
96
]
},
{
"text": "SarfarazAhmed",
"indices": [
97,
111
]
},
{
"text": "jasonroy",
"indices": [
112,
121
]
}
],
"symbols": [],
"user_mentions": [
{
"screen_name": "Ayemanmalik01",
"name": "Ayemanmalik01\ud83c\uddf5\ud83c\uddf0",
"id": 1138458135625109509,
"id_str": "1138458135625109509",
"indices": [
3,
17
]
}
],
"urls": []
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1111759698942939144,
"id_str": "1111759698942939144",
"name": "Maha Malik98 \u270c\ufe0f",
"screen_name": "maha_malik98",
"location": "Karachi ",
"description": "#Cricket lover\n#Supporter of Karachi Kings \ud83d\udc51\n#Imadian\n#favorite cricketer @simadwasim\n#Capricorn\n#iBleedGreen.. \ud83c\uddf5\ud83c\uddf0\ud83d\udc9a\nLead the man be the change",
"url": "https://t.co/pylQtwry2u",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/pylQtwry2u",
"expanded_url": "http://instagram.com/lmadwasim09",
"display_url": "instagram.com/lmadwasim09",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 303,
"friends_count": 78,
"listed_count": 1,
"created_at": "Fri Mar 29 22:39:02 +0000 2019",
"favourites_count": 16321,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 6678,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1328454761557987328/hk4EkfzM_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1328454761557987328/hk4EkfzM_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1111759698942939144/1565472543",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"retweeted_status": {
"created_at": "Mon Feb 07 17:14:48 +0000 2022",
"id": 1490735809036705795,
"id_str": "1490735809036705795",
"text": "Roy oh My Boyyy\ud83d\udd25\ud83e\udd2f\ud83e\udd29What an Innings BrOooooo\ud83e\udd18\ud83d\ude4c\n\n#LQvQG #QGvLQ #Cricket #PSL2022 #SarfarazAhmed #jasonroy https://t.co/dGvipRFRZZ",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "LQvQG",
"indices": [
46,
52
]
},
{
"text": "QGvLQ",
"indices": [
53,
59
]
},
{
"text": "Cricket",
"indices": [
60,
68
]
},
{
"text": "PSL2022",
"indices": [
69,
77
]
},
{
"text": "SarfarazAhmed",
"indices": [
78,
92
]
},
{
"text": "jasonroy",
"indices": [
93,
102
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490735773565472770,
"id_str": "1490735773565472770",
"indices": [
103,
126
],
"media_url": "http://pbs.twimg.com/media/FLAoSa1WUAIvd3O.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLAoSa1WUAIvd3O.jpg",
"url": "https://t.co/dGvipRFRZZ",
"display_url": "pic.twitter.com/dGvipRFRZZ",
"expanded_url": "https://twitter.com/Ayemanmalik01/status/1490735809036705795/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"small": {
"w": 454,
"h": 680,
"resize": "fit"
},
"large": {
"w": 1366,
"h": 2048,
"resize": "fit"
},
"medium": {
"w": 800,
"h": 1200,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490735773565472770,
"id_str": "1490735773565472770",
"indices": [
103,
126
],
"media_url": "http://pbs.twimg.com/media/FLAoSa1WUAIvd3O.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLAoSa1WUAIvd3O.jpg",
"url": "https://t.co/dGvipRFRZZ",
"display_url": "pic.twitter.com/dGvipRFRZZ",
"expanded_url": "https://twitter.com/Ayemanmalik01/status/1490735809036705795/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"small": {
"w": 454,
"h": 680,
"resize": "fit"
},
"large": {
"w": 1366,
"h": 2048,
"resize": "fit"
},
"medium": {
"w": 800,
"h": 1200,
"resize": "fit"
}
}
},
{
"id": 1490735792918048773,
"id_str": "1490735792918048773",
"indices": [
103,
126
],
"media_url": "http://pbs.twimg.com/media/FLAoTi7XMAU4nsv.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLAoTi7XMAU4nsv.jpg",
"url": "https://t.co/dGvipRFRZZ",
"display_url": "pic.twitter.com/dGvipRFRZZ",
"expanded_url": "https://twitter.com/Ayemanmalik01/status/1490735809036705795/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 799,
"h": 1200,
"resize": "fit"
},
"large": {
"w": 1364,
"h": 2048,
"resize": "fit"
},
"small": {
"w": 453,
"h": 680,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1138458135625109509,
"id_str": "1138458135625109509",
"name": "Ayemanmalik01\ud83c\uddf5\ud83c\uddf0",
"screen_name": "Ayemanmalik01",
"location": "Cricket World \ud83c\udf0d",
"description": "No DM Allow\u274c\n#Cricket Freak\ud83c\uddf5\ud83c\uddf0\nSUPPORTER of #BabarAzam & @sarfarazA_54\n\n\n#Alhamdullilah Muslim\ud83d\ude07\n\nGot likes from #Sarfaraz, #Amir , #Wahab & #Shoaib Malik\u2665\ufe0f",
"url": "https://t.co/oCQ3mN2EKq",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/oCQ3mN2EKq",
"expanded_url": "http://www.instagram.com/ayemanmalik01",
"display_url": "instagram.com/ayemanmalik01",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 2199,
"friends_count": 66,
"listed_count": 0,
"created_at": "Tue Jun 11 14:49:05 +0000 2019",
"favourites_count": 28080,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 12466,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1424708573280542720/HdzhUiOH_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1424708573280542720/HdzhUiOH_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1138458135625109509/1621723611",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 4,
"favorite_count": 8,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
"is_quote_status": false,
"retweet_count": 4,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:34 +0000 2022",
"id": 1490754879140372480,
"id_str": "1490754879140372480",
"text": "RT @geonews_urdu: 205 \u0631\u0646\u0632 \u06a9\u0627 \u06c1\u062f\u0641 \u06a9\u0648\u0626\u0679\u06c1 \u0646\u06d2 20 \u0648\u06cc\u06ba \u0627\u0648\u0648\u0631 \u0645\u06cc\u06ba \u0645\u06a9\u0645\u0644 \u06a9\u0631\u0644\u06cc\u0627\u060c \u062c\u06cc\u0633\u0646 \u0631\u0648\u0626\u06d2 57 \u06af\u06cc\u0646\u062f\u0648\u06ba \u067e\u0631 116 \u0631\u0646\u0632 \u0628\u0646\u0627\u06a9\u0631 \u0646\u0645\u0627\u06cc\u0627\u06ba \u0631\u06c1\u06d2\n \u0645\u0632\u06cc\u062f \u062c\u0627\u0646\u06cc\u06d2 :https://\u2026",
"truncated": false,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [
{
"screen_name": "geonews_urdu",
"name": "Geo News Urdu",
"id": 165796189,
"id_str": "165796189",
"indices": [
3,
16
]
}
],
"urls": []
},
"metadata": {
"iso_language_code": "ur",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1158808385959157762,
"id_str": "1158808385959157762",
"name": "WardahRafiq\ud83d\udc9c",
"screen_name": "PAKARMYBTSBANG",
"location": "Pakistan",
"description": "BTS AND BTS..\n\ud83d\udc9c\ud83d\udc9c\nBTS ARMY..\nTaehyung Jungkook\ud83d\udc95\nPROUD PAKISTANI...\ud83c\uddf5\ud83c\uddf0",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 101,
"friends_count": 432,
"listed_count": 1,
"created_at": "Tue Aug 06 18:33:43 +0000 2019",
"favourites_count": 19701,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 4311,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1457466341619798018/2zpg4-7g_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1457466341619798018/2zpg4-7g_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1158808385959157762/1623505231",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"retweeted_status": {
"created_at": "Mon Feb 07 18:30:18 +0000 2022",
"id": 1490754811649982464,
"id_str": "1490754811649982464",
"text": "205 \u0631\u0646\u0632 \u06a9\u0627 \u06c1\u062f\u0641 \u06a9\u0648\u0626\u0679\u06c1 \u0646\u06d2 20 \u0648\u06cc\u06ba \u0627\u0648\u0648\u0631 \u0645\u06cc\u06ba \u0645\u06a9\u0645\u0644 \u06a9\u0631\u0644\u06cc\u0627\u060c \u062c\u06cc\u0633\u0646 \u0631\u0648\u0626\u06d2 57 \u06af\u06cc\u0646\u062f\u0648\u06ba \u067e\u0631 116 \u0631\u0646\u0632 \u0628\u0646\u0627\u06a9\u0631 \u0646\u0645\u0627\u06cc\u0627\u06ba \u0631\u06c1\u06d2\n \u0645\u0632\u06cc\u062f \u062c\u0627\u0646\u06cc\u06d2 :\u2026 https://t.co/qd7vfcsNmH",
"truncated": true,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [],
"urls": [
{
"url": "https://t.co/qd7vfcsNmH",
"expanded_url": "https://twitter.com/i/web/status/1490754811649982464",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
115,
138
]
}
]
},
"metadata": {
"iso_language_code": "ur",
"result_type": "recent"
},
"source": "<a href=\"https://about.twitter.com/products/tweetdeck\" rel=\"nofollow\">TweetDeck</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 165796189,
"id_str": "165796189",
"name": "Geo News Urdu",
"screen_name": "geonews_urdu",
"location": "Pakistan",
"description": "Geo is Pakistan's No. 1 TV Channel catering latest local, international, sports, business and entertainment news round the clock. | Instagram: geourdudottv",
"url": "https://t.co/GBqKsuXyH8",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/GBqKsuXyH8",
"expanded_url": "https://urdu.geo.tv/",
"display_url": "urdu.geo.tv",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 3953665,
"friends_count": 11,
"listed_count": 2166,
"created_at": "Mon Jul 12 15:24:32 +0000 2010",
"favourites_count": 0,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": true,
"statuses_count": 3348221,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1300144278526734336/W4V2QH7Q_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1300144278526734336/W4V2QH7Q_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/165796189/1610733680",
"profile_link_color": "B22222",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": false,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "regular",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 2,
"favorite_count": 76,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "ur"
},
"is_quote_status": false,
"retweet_count": 2,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "ur"
},
{
"created_at": "Mon Feb 07 18:30:33 +0000 2022",
"id": 1490754874228854788,
"id_str": "1490754874228854788",
"text": "Jason roy we will see u in Lahore \ud83d\udc94\ud83e\udd1d \n@JasonRoy20 #PSL7 #LQvsQG #Jasonroy #QGvLQ https://t.co/u7OJYnHedx",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "PSL7",
"indices": [
50,
55
]
},
{
"text": "LQvsQG",
"indices": [
56,
63
]
},
{
"text": "Jasonroy",
"indices": [
64,
73
]
},
{
"text": "QGvLQ",
"indices": [
74,
80
]
}
],
"symbols": [],
"user_mentions": [
{
"screen_name": "JasonRoy20",
"name": "Jason Roy",
"id": 343512172,
"id_str": "343512172",
"indices": [
38,
49
]
}
],
"urls": [],
"media": [
{
"id": 1490754865987047426,
"id_str": "1490754865987047426",
"indices": [
81,
104
],
"media_url": "http://pbs.twimg.com/media/FLA5pvpX0AISP1C.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5pvpX0AISP1C.jpg",
"url": "https://t.co/u7OJYnHedx",
"display_url": "pic.twitter.com/u7OJYnHedx",
"expanded_url": "https://twitter.com/nasir_sheharyar/status/1490754874228854788/photo/1",
"type": "photo",
"sizes": {
"large": {
"w": 1242,
"h": 1045,
"resize": "fit"
},
"small": {
"w": 680,
"h": 572,
"resize": "fit"
},
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 1010,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754865987047426,
"id_str": "1490754865987047426",
"indices": [
81,
104
],
"media_url": "http://pbs.twimg.com/media/FLA5pvpX0AISP1C.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5pvpX0AISP1C.jpg",
"url": "https://t.co/u7OJYnHedx",
"display_url": "pic.twitter.com/u7OJYnHedx",
"expanded_url": "https://twitter.com/nasir_sheharyar/status/1490754874228854788/photo/1",
"type": "photo",
"sizes": {
"large": {
"w": 1242,
"h": 1045,
"resize": "fit"
},
"small": {
"w": 680,
"h": 572,
"resize": "fit"
},
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 1010,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/iphone\" rel=\"nofollow\">Twitter for iPhone</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1270307535728381957,
"id_str": "1270307535728381957",
"name": "oye.sherry Tweets || LQ\ud83d\udc9a||",
"screen_name": "nasir_sheharyar",
"location": "Lahore, Pakistan",
"description": "{ WORK HARD UNTIL YOUR IDOLS BECOME YOUR RIVALS }\u201c\ud83d\ude08\u201d \ud83d\udc49\ud83c\udffb\ud83c\udfcf\u2764\ufe0f\ud83d\udc48\ud83c\udffb",
"url": "https://t.co/xd2fjXrqhp",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/xd2fjXrqhp",
"expanded_url": "https://instagram.com/m.sherry._11?igshid=1aj76dwudde6s",
"display_url": "instagram.com/m.sherry._11?i\u2026",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 125,
"friends_count": 246,
"listed_count": 1,
"created_at": "Tue Jun 09 10:51:45 +0000 2020",
"favourites_count": 10321,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 927,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1479523931023360008/dfVQKbZF_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1479523931023360008/dfVQKbZF_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1270307535728381957/1641580944",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 3,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:31 +0000 2022",
"id": 1490754867668918278,
"id_str": "1490754867668918278",
"text": "Unbeatable innings by #JasonRoy...\nYou played like a real gladiator today mann!!!\ud83d\udc4f\ud83d\udc4f\n#lQvsQg #PSL7 #MainHoonQalandar https://t.co/vMymUbi8Zy",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "JasonRoy",
"indices": [
22,
31
]
},
{
"text": "lQvsQg",
"indices": [
84,
91
]
},
{
"text": "PSL7",
"indices": [
92,
97
]
},
{
"text": "MainHoonQalandar",
"indices": [
98,
115
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490754865022263297,
"id_str": "1490754865022263297",
"indices": [
116,
139
],
"media_url": "http://pbs.twimg.com/media/FLA5psDWYAEsp7c.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5psDWYAEsp7c.jpg",
"url": "https://t.co/vMymUbi8Zy",
"display_url": "pic.twitter.com/vMymUbi8Zy",
"expanded_url": "https://twitter.com/HafsaAbdulRehm5/status/1490754867668918278/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1078,
"h": 595,
"resize": "fit"
},
"small": {
"w": 680,
"h": 375,
"resize": "fit"
},
"large": {
"w": 1078,
"h": 595,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754865022263297,
"id_str": "1490754865022263297",
"indices": [
116,
139
],
"media_url": "http://pbs.twimg.com/media/FLA5psDWYAEsp7c.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5psDWYAEsp7c.jpg",
"url": "https://t.co/vMymUbi8Zy",
"display_url": "pic.twitter.com/vMymUbi8Zy",
"expanded_url": "https://twitter.com/HafsaAbdulRehm5/status/1490754867668918278/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1078,
"h": 595,
"resize": "fit"
},
"small": {
"w": 680,
"h": 375,
"resize": "fit"
},
"large": {
"w": 1078,
"h": 595,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1364213213210927111,
"id_str": "1364213213210927111",
"name": "Little_lioness\ud83e\udd81\u2728",
"screen_name": "HafsaAbdulRehm5",
"location": "",
"description": "Proud to be a Pakistani Muslim \u2764\ufe0f\ud83c\uddf5\ud83c\uddf0\nI can&I will\u270c\ufe0f\u2764\ufe0f",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 16,
"friends_count": 59,
"listed_count": 0,
"created_at": "Tue Feb 23 13:59:39 +0000 2021",
"favourites_count": 217,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 187,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1364213762216103936/yEm-ZdTZ_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1364213762216103936/yEm-ZdTZ_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1364213213210927111/1644228767",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 1,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:30 +0000 2022",
"id": 1490754861000019970,
"id_str": "1490754861000019970",
"text": "Boom Afridi.\n#PSL2022 #jasonRoy #QGvLQ https://t.co/LMPqruwDku",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "PSL2022",
"indices": [
13,
21
]
},
{
"text": "jasonRoy",
"indices": [
22,
31
]
},
{
"text": "QGvLQ",
"indices": [
32,
38
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490754848354185221,
"id_str": "1490754848354185221",
"indices": [
39,
62
],
"media_url": "http://pbs.twimg.com/media/FLA5ot9XsAUMIci.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5ot9XsAUMIci.jpg",
"url": "https://t.co/LMPqruwDku",
"display_url": "pic.twitter.com/LMPqruwDku",
"expanded_url": "https://twitter.com/HarisMa73708878/status/1490754861000019970/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"small": {
"w": 680,
"h": 379,
"resize": "fit"
},
"large": {
"w": 1242,
"h": 692,
"resize": "fit"
},
"medium": {
"w": 1200,
"h": 669,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754848354185221,
"id_str": "1490754848354185221",
"indices": [
39,
62
],
"media_url": "http://pbs.twimg.com/media/FLA5ot9XsAUMIci.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5ot9XsAUMIci.jpg",
"url": "https://t.co/LMPqruwDku",
"display_url": "pic.twitter.com/LMPqruwDku",
"expanded_url": "https://twitter.com/HarisMa73708878/status/1490754861000019970/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"small": {
"w": 680,
"h": 379,
"resize": "fit"
},
"large": {
"w": 1242,
"h": 692,
"resize": "fit"
},
"medium": {
"w": 1200,
"h": 669,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1058187070370443271,
"id_str": "1058187070370443271",
"name": "Haris Mahmood",
"screen_name": "HarisMa73708878",
"location": "Sargodha, Pakistan",
"description": "fan of boom boom afridi...\ud83d\udcaa\ud83d\udcaa?\nfollow me on Instagram..https://t.co/NcqMCwDxMJ\nmy youtube channel.https://t.co/NCEpy0uJWb",
"url": null,
"entities": {
"description": {
"urls": [
{
"url": "https://t.co/NcqMCwDxMJ",
"expanded_url": "https://www.instagram.com/harismahmoodofficia",
"display_url": "instagram.com/harismahmoodof\u2026",
"indices": [
54,
77
]
},
{
"url": "https://t.co/NCEpy0uJWb",
"expanded_url": "https://youtu.be/UP4ys8CRKmE",
"display_url": "youtu.be/UP4ys8CRKmE",
"indices": [
97,
120
]
}
]
}
},
"protected": false,
"followers_count": 1403,
"friends_count": 2138,
"listed_count": 0,
"created_at": "Fri Nov 02 02:40:32 +0000 2018",
"favourites_count": 63265,
"utc_offset": null,
"time_zone": null,
"geo_enabled": true,
"verified": false,
"statuses_count": 9256,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1456648507906011136/JAUaHxpU_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1456648507906011136/JAUaHxpU_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1058187070370443271/1630592728",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:18 +0000 2022",
"id": 1490754811649982464,
"id_str": "1490754811649982464",
"text": "205 \u0631\u0646\u0632 \u06a9\u0627 \u06c1\u062f\u0641 \u06a9\u0648\u0626\u0679\u06c1 \u0646\u06d2 20 \u0648\u06cc\u06ba \u0627\u0648\u0648\u0631 \u0645\u06cc\u06ba \u0645\u06a9\u0645\u0644 \u06a9\u0631\u0644\u06cc\u0627\u060c \u062c\u06cc\u0633\u0646 \u0631\u0648\u0626\u06d2 57 \u06af\u06cc\u0646\u062f\u0648\u06ba \u067e\u0631 116 \u0631\u0646\u0632 \u0628\u0646\u0627\u06a9\u0631 \u0646\u0645\u0627\u06cc\u0627\u06ba \u0631\u06c1\u06d2\n \u0645\u0632\u06cc\u062f \u062c\u0627\u0646\u06cc\u06d2 :\u2026 https://t.co/qd7vfcsNmH",
"truncated": true,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [],
"urls": [
{
"url": "https://t.co/qd7vfcsNmH",
"expanded_url": "https://twitter.com/i/web/status/1490754811649982464",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
115,
138
]
}
]
},
"metadata": {
"iso_language_code": "ur",
"result_type": "recent"
},
"source": "<a href=\"https://about.twitter.com/products/tweetdeck\" rel=\"nofollow\">TweetDeck</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 165796189,
"id_str": "165796189",
"name": "Geo News Urdu",
"screen_name": "geonews_urdu",
"location": "Pakistan",
"description": "Geo is Pakistan's No. 1 TV Channel catering latest local, international, sports, business and entertainment news round the clock. | Instagram: geourdudottv",
"url": "https://t.co/GBqKsuXyH8",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/GBqKsuXyH8",
"expanded_url": "https://urdu.geo.tv/",
"display_url": "urdu.geo.tv",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 3953665,
"friends_count": 11,
"listed_count": 2166,
"created_at": "Mon Jul 12 15:24:32 +0000 2010",
"favourites_count": 0,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": true,
"statuses_count": 3348221,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1300144278526734336/W4V2QH7Q_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1300144278526734336/W4V2QH7Q_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/165796189/1610733680",
"profile_link_color": "B22222",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": false,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "regular",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 2,
"favorite_count": 76,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "ur"
},
{
"created_at": "Mon Feb 07 18:30:13 +0000 2022",
"id": 1490754790481358848,
"id_str": "1490754790481358848",
"text": "Two vital points for Quetta Gladiators.\n\n#PSL2022 #LahoreQalandars #QuettaGladiators #Cricket #JasonRoy #BetHive https://t.co/ICU16Tky94",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "PSL2022",
"indices": [
41,
49
]
},
{
"text": "LahoreQalandars",
"indices": [
50,
66
]
},
{
"text": "QuettaGladiators",
"indices": [
67,
84
]
},
{
"text": "Cricket",
"indices": [
85,
93
]
},
{
"text": "JasonRoy",
"indices": [
94,
103
]
},
{
"text": "BetHive",
"indices": [
104,
112
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490754776199725056,
"id_str": "1490754776199725056",
"indices": [
113,
136
],
"media_url": "http://pbs.twimg.com/media/FLA5khKaAAAUhNP.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5khKaAAAUhNP.jpg",
"url": "https://t.co/ICU16Tky94",
"display_url": "pic.twitter.com/ICU16Tky94",
"expanded_url": "https://twitter.com/The_BetHive/status/1490754790481358848/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 1200,
"resize": "fit"
},
"small": {
"w": 680,
"h": 680,
"resize": "fit"
},
"large": {
"w": 1400,
"h": 1400,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754776199725056,
"id_str": "1490754776199725056",
"indices": [
113,
136
],
"media_url": "http://pbs.twimg.com/media/FLA5khKaAAAUhNP.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5khKaAAAUhNP.jpg",
"url": "https://t.co/ICU16Tky94",
"display_url": "pic.twitter.com/ICU16Tky94",
"expanded_url": "https://twitter.com/The_BetHive/status/1490754790481358848/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"medium": {
"w": 1200,
"h": 1200,
"resize": "fit"
},
"small": {
"w": 680,
"h": 680,
"resize": "fit"
},
"large": {
"w": 1400,
"h": 1400,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"https://mobile.twitter.com\" rel=\"nofollow\">Twitter Web App</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1346153776999239683,
"id_str": "1346153776999239683",
"name": "BetHive",
"screen_name": "The_BetHive",
"location": "",
"description": "The Hive of Sports. Get all the latest sports content.",
"url": "https://t.co/knpsbALNBt",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/knpsbALNBt",
"expanded_url": "https://bethive.net/",
"display_url": "bethive.net",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 48,
"friends_count": 0,
"listed_count": 1,
"created_at": "Mon Jan 04 17:57:34 +0000 2021",
"favourites_count": 0,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 2353,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1377889762418196483/9pHsi8Az_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1377889762418196483/9pHsi8Az_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1346153776999239683/1643700377",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:08 +0000 2022",
"id": 1490754770067464196,
"id_str": "1490754770067464196",
"text": "Jason Dhoye \nLahore Roy \ud83e\udd72 \n#LQvsQG #QGvsLQ #PSL7 #JasonRoy #lqvsqg https://t.co/HccWvoCQ88",
"truncated": false,
"entities": {
"hashtags": [
{
"text": "LQvsQG",
"indices": [
27,
34
]
},
{
"text": "QGvsLQ",
"indices": [
35,
42
]
},
{
"text": "PSL7",
"indices": [
43,
48
]
},
{
"text": "JasonRoy",
"indices": [
49,
58
]
},
{
"text": "lqvsqg",
"indices": [
59,
66
]
}
],
"symbols": [],
"user_mentions": [],
"urls": [],
"media": [
{
"id": 1490754764413587457,
"id_str": "1490754764413587457",
"indices": [
67,
90
],
"media_url": "http://pbs.twimg.com/media/FLA5j1QX0AENHlp.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5j1QX0AENHlp.jpg",
"url": "https://t.co/HccWvoCQ88",
"display_url": "pic.twitter.com/HccWvoCQ88",
"expanded_url": "https://twitter.com/nthin2u/status/1490754770067464196/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"large": {
"w": 350,
"h": 200,
"resize": "fit"
},
"small": {
"w": 350,
"h": 200,
"resize": "fit"
},
"medium": {
"w": 350,
"h": 200,
"resize": "fit"
}
}
}
]
},
"extended_entities": {
"media": [
{
"id": 1490754764413587457,
"id_str": "1490754764413587457",
"indices": [
67,
90
],
"media_url": "http://pbs.twimg.com/media/FLA5j1QX0AENHlp.jpg",
"media_url_https": "https://pbs.twimg.com/media/FLA5j1QX0AENHlp.jpg",
"url": "https://t.co/HccWvoCQ88",
"display_url": "pic.twitter.com/HccWvoCQ88",
"expanded_url": "https://twitter.com/nthin2u/status/1490754770067464196/photo/1",
"type": "photo",
"sizes": {
"thumb": {
"w": 150,
"h": 150,
"resize": "crop"
},
"large": {
"w": 350,
"h": 200,
"resize": "fit"
},
"small": {
"w": 350,
"h": 200,
"resize": "fit"
},
"medium": {
"w": 350,
"h": 200,
"resize": "fit"
}
}
}
]
},
"metadata": {
"iso_language_code": "es",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/iphone\" rel=\"nofollow\">Twitter for iPhone</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1303238503254437888,
"id_str": "1303238503254437888",
"name": "Hafsa Shahid Awan",
"screen_name": "nthin2u",
"location": "Lahore, Pakistan",
"description": "\u0648\u0642\u062a \u06a9\u0648 \u0628\u06be\u06cc \u062a\u06be\u0648\u0691\u0627 \u0648\u0642\u062a \u062f\u0648",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 2,
"friends_count": 6,
"listed_count": 0,
"created_at": "Tue Sep 08 07:49:06 +0000 2020",
"favourites_count": 15,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 4,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1303239348255633409/PzAdJiTS_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1303239348255633409/PzAdJiTS_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1303238503254437888/1599552553",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 2,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "es"
},
{
"created_at": "Mon Feb 07 18:30:05 +0000 2022",
"id": 1490754757253898245,
"id_str": "1490754757253898245",
"text": "RT @CricksLab: 'He Came, He Saw, He Conquered' \n\n@JasonRoy20 giving high hopes to the Quetta Gladiators as they beat Lahore Qalandars by 7\u2026",
"truncated": false,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [
{
"screen_name": "CricksLab",
"name": "CricksLab",
"id": 950368378182688768,
"id_str": "950368378182688768",
"indices": [
3,
13
]
},
{
"screen_name": "JasonRoy20",
"name": "Jason Roy",
"id": 343512172,
"id_str": "343512172",
"indices": [
49,
60
]
}
],
"urls": []
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 1355183662258663429,
"id_str": "1355183662258663429",
"name": "bhatak_rahi_hn\ud83d\udc40(KK\ud83d\udc99)",
"screen_name": "Bhatkti_atma1",
"location": "Karachi, Pakistan",
"description": "",
"url": null,
"entities": {
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 19,
"friends_count": 15,
"listed_count": 0,
"created_at": "Fri Jan 29 15:59:20 +0000 2021",
"favourites_count": 682,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 426,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1489521191698288640/Ymc9DRDE_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1489521191698288640/Ymc9DRDE_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1355183662258663429/1644163657",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"retweeted_status": {
"created_at": "Mon Feb 07 18:26:46 +0000 2022",
"id": 1490753922767618049,
"id_str": "1490753922767618049",
"text": "'He Came, He Saw, He Conquered' \n\n@JasonRoy20 giving high hopes to the Quetta Gladiators as they beat Lahore Qaland\u2026 https://t.co/ZFbKmo3EmZ",
"truncated": true,
"entities": {
"hashtags": [],
"symbols": [],
"user_mentions": [
{
"screen_name": "JasonRoy20",
"name": "Jason Roy",
"id": 343512172,
"id_str": "343512172",
"indices": [
34,
45
]
}
],
"urls": [
{
"url": "https://t.co/ZFbKmo3EmZ",
"expanded_url": "https://twitter.com/i/web/status/1490753922767618049",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
117,
140
]
}
]
},
"metadata": {
"iso_language_code": "en",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 950368378182688768,
"id_str": "950368378182688768",
"name": "CricksLab",
"screen_name": "CricksLab",
"location": "Dubai, UAE",
"description": "The World's # 1 Cricket Management Platform | Live Scores | HD Graphics | Live Streaming | Player Auction Module | Team Profiles | Advance Insights | Stats |",
"url": "https://t.co/GG05sAFs48",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/GG05sAFs48",
"expanded_url": "https://crickslab.com",
"display_url": "crickslab.com",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 1316,
"friends_count": 28,
"listed_count": 2,
"created_at": "Mon Jan 08 14:07:33 +0000 2018",
"favourites_count": 32,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 446,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "000000",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1486659742965673985/zHQ-RMBm_normal.png",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1486659742965673985/zHQ-RMBm_normal.png",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/950368378182688768/1643282297",
"profile_link_color": "1B95E0",
"profile_sidebar_border_color": "000000",
"profile_sidebar_fill_color": "000000",
"profile_text_color": "000000",
"profile_use_background_image": false,
"has_extended_profile": false,
"default_profile": false,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 7,
"favorite_count": 68,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "en"
},
"is_quote_status": false,
"retweet_count": 7,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "en"
},
{
"created_at": "Mon Feb 07 18:30:02 +0000 2022",
"id": 1490754745958608900,
"id_str": "1490754745958608900",
"text": "@thePSLt20 Saifi Bhai match ke baad Jason Roy ko Bufferzone ki galliyon ka chakkar dilanay le jaatay hoye #PSL7\u2026 https://t.co/ewsF29KOqD",
"truncated": true,
"entities": {
"hashtags": [
{
"text": "PSL7",
"indices": [
106,
111
]
}
],
"symbols": [],
"user_mentions": [
{
"screen_name": "thePSLt20",
"name": "PakistanSuperLeague",
"id": 3448716797,
"id_str": "3448716797",
"indices": [
0,
10
]
}
],
"urls": [
{
"url": "https://t.co/ewsF29KOqD",
"expanded_url": "https://twitter.com/i/web/status/1490754745958608900",
"display_url": "twitter.com/i/web/status/1\u2026",
"indices": [
113,
136
]
}
]
},
"metadata": {
"iso_language_code": "hi",
"result_type": "recent"
},
"source": "<a href=\"http://twitter.com/download/android\" rel=\"nofollow\">Twitter for Android</a>",
"in_reply_to_status_id": 1490754219187527682,
"in_reply_to_status_id_str": "1490754219187527682",
"in_reply_to_user_id": 3448716797,
"in_reply_to_user_id_str": "3448716797",
"in_reply_to_screen_name": "thePSLt20",
"user": {
"id": 1028682454373224450,
"id_str": "1028682454373224450",
"name": "Ismaeel Rana",
"screen_name": "IsmaeelRana31",
"location": "Punjab, Pakistan",
"description": "Muslim , Pakistani , Rajput",
"url": "https://t.co/yRD46HnpAq",
"entities": {
"url": {
"urls": [
{
"url": "https://t.co/yRD46HnpAq",
"expanded_url": "http://Www.Ismaeelrana31.com.pk",
"display_url": "Ismaeelrana31.com.pk",
"indices": [
0,
23
]
}
]
},
"description": {
"urls": []
}
},
"protected": false,
"followers_count": 202,
"friends_count": 49,
"listed_count": 0,
"created_at": "Sun Aug 12 16:39:43 +0000 2018",
"favourites_count": 5849,
"utc_offset": null,
"time_zone": null,
"geo_enabled": false,
"verified": false,
"statuses_count": 1480,
"lang": null,
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "F5F8FA",
"profile_background_image_url": null,
"profile_background_image_url_https": null,
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/1486932235886473216/snnbWnxG_normal.jpg",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/1486932235886473216/snnbWnxG_normal.jpg",
"profile_banner_url": "https://pbs.twimg.com/profile_banners/1028682454373224450/1643347399",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": true,
"default_profile": true,
"default_profile_image": false,
"following": false,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none",
"withheld_in_countries": []
},
"geo": null,
"coordinates": null,
"place": null,
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 2,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"lang": "hi"
}
]
len(statuses)
200
json.dumps(statuses[1])
'{"created_at": "Mon Feb 07 19:18:37 +0000 2022", "id": 1490766968294682625, "id_str": "1490766968294682625", "text": "SHEHRI DEHLEEZ PR MEHFUZ NHI | C110NEWS HD | REPORTED BY TAIMOOR SHAKIL \\n\\n#C110NEWS #JasonRoy #QGvsLQ #LQvQG #KKvIU\\u2026 https://t.co/2ZpEmFwW5v", "truncated": true, "entities": {"hashtags": [{"text": "C110NEWS", "indices": [74, 83]}, {"text": "JasonRoy", "indices": [84, 93]}, {"text": "QGvsLQ", "indices": [94, 101]}, {"text": "LQvQG", "indices": [102, 108]}, {"text": "KKvIU", "indices": [109, 115]}], "symbols": [], "user_mentions": [], "urls": [{"url": "https://t.co/2ZpEmFwW5v", "expanded_url": "https://twitter.com/i/web/status/1490766968294682625", "display_url": "twitter.com/i/web/status/1\\u2026", "indices": [117, 140]}]}, "metadata": {"iso_language_code": "en", "result_type": "recent"}, "source": "<a href=\\"https://mobile.twitter.com\\" rel=\\"nofollow\\">Twitter Web App</a>", "in_reply_to_status_id": null, "in_reply_to_status_id_str": null, "in_reply_to_user_id": null, "in_reply_to_user_id_str": null, "in_reply_to_screen_name": null, "user": {"id": 1202592531713798144, "id_str": "1202592531713798144", "name": "C110 News HD", "screen_name": "C110NewsHD", "location": "Karachi, Pakistan", "description": "", "url": "https://t.co/SUX389ajHu", "entities": {"url": {"urls": [{"url": "https://t.co/SUX389ajHu", "expanded_url": "http://www.c110news.tv", "display_url": "c110news.tv", "indices": [0, 23]}]}, "description": {"urls": []}}, "protected": false, "followers_count": 30, "friends_count": 0, "listed_count": 1, "created_at": "Thu Dec 05 14:16:26 +0000 2019", "favourites_count": 2, "utc_offset": null, "time_zone": null, "geo_enabled": false, "verified": false, "statuses_count": 4378, "lang": null, "contributors_enabled": false, "is_translator": false, "is_translation_enabled": false, "profile_background_color": "F5F8FA", "profile_background_image_url": null, "profile_background_image_url_https": null, "profile_background_tile": false, "profile_image_url": "http://pbs.twimg.com/profile_images/1202593758447095808/Ddfz-mca_normal.jpg", "profile_image_url_https": "https://pbs.twimg.com/profile_images/1202593758447095808/Ddfz-mca_normal.jpg", "profile_banner_url": "https://pbs.twimg.com/profile_banners/1202592531713798144/1575560437", "profile_link_color": "1DA1F2", "profile_sidebar_border_color": "C0DEED", "profile_sidebar_fill_color": "DDEEF6", "profile_text_color": "333333", "profile_use_background_image": true, "has_extended_profile": false, "default_profile": true, "default_profile_image": false, "following": false, "follow_request_sent": false, "notifications": false, "translator_type": "none", "withheld_in_countries": []}, "geo": null, "coordinates": null, "place": null, "contributors": null, "is_quote_status": false, "retweet_count": 0, "favorite_count": 0, "favorited": false, "retweeted": false, "possibly_sensitive": false, "lang": "en"}'
Creating a basic frequency distribution from the words in tweets#
from collections import Counter
for item in [words, screen_names, hashtags]:
c = Counter(item)
print(c.most_common()[:10]) # top 10
[('#JasonRoy', 86), ('the', 64), ('RT', 59), ('a', 43), ('Jason', 41), ('#QGvsLQ', 34), ('of', 34), ('#jasonroy', 33), ('in', 32), ('#QGvLQ', 32)]
[('JasonRoy20', 29), ('TeamQuetta', 18), ('lahoreqalandars', 9), ('Ayemanmalik01', 7), ('thePSLt20', 7), ('CricMady', 5), ('SarfarazA_54', 4), ('CricksLab', 4), ('arynewsud', 3), ('NaikRooh', 3)]
[('JasonRoy', 87), ('PSL7', 37), ('QGvsLQ', 36), ('jasonroy', 34), ('QGvLQ', 34), ('PSL2022', 22), ('LQvQG', 20), ('Cricket', 13), ('Jasonroy', 11), ('QuettaGladiators', 11)]
words
['Alhamdulillah!!',
'What',
'a',
'win',
'💜💜',
'Yahi',
'comeback',
'chahiye',
'tha',
'saifi',
'Bhai',
'.',
'IN',
'SHA',
'ALLAH',
'Trophy',
'bhi',
'Quetta',
'gladiators',
'ki…',
'https://t.co/aCgpGAiLHW',
'SHEHRI',
'DEHLEEZ',
'PR',
'MEHFUZ',
'NHI',
'|',
'C110NEWS',
'HD',
'|',
'REPORTED',
'BY',
'TAIMOOR',
'SHAKIL',
'#C110NEWS',
'#JasonRoy',
'#QGvsLQ',
'#LQvQG',
'#KKvIU…',
'https://t.co/2ZpEmFwW5v',
'𝐓𝐡𝐚𝐧𝐤',
'𝐲𝐨𝐮',
'#𝐊𝐚𝐫𝐚𝐜𝐡𝐢',
'𝐟𝐨𝐫',
'𝐞𝐧𝐭𝐞𝐫𝐭𝐚𝐢𝐧𝐢𝐧𝐠',
'𝐮𝐬¡',
'What',
'a',
'game',
'of',
'cricket',
'between',
'#QuettaGladitors',
'&',
'#LahoreQalandars,',
'so',
'aggr…',
'https://t.co/zGDvcne1hu',
'I',
'was',
'supporting',
'@lahoreqalandars',
'But',
'the',
'way',
'@JasonRoy20',
'bat',
'That',
'was',
'just',
'superb👏👏👏',
'One',
'of',
'my',
'favorites',
'❤️❤️❤️❤️…',
'https://t.co/VJd3ytZ46j',
'RT',
'@SarfarazA_Fan:',
'روئے',
'آپ',
'کی',
'وجہ',
'سے',
'آج',
'لاہوری',
'بہت',
'روئے',
'ہیں',
'۔😉😉',
'Congratulations',
'@TeamQuetta',
'@SarfarazA_54',
'💜❤️',
'very',
'well',
'played',
'@JasonRoy20…',
'RT',
'@Ayemanmalik01:',
'You',
'can',
'Either',
'be',
'A',
'Hero',
'or',
'Ex',
'with',
'no',
'Dignity',
'😂💯🤩',
'#LQvQG',
'#Cricket',
'#JasonRoy',
'#PSL2022',
'#SarfarazAhmed',
'#ShaheenShahAfridi…',
'RT',
'@SarfarazA_Fan:',
'روئے',
'آپ',
'کی',
'وجہ',
'سے',
'آج',
'لاہوری',
'بہت',
'روئے',
'ہیں',
'۔😉😉',
'Congratulations',
'@TeamQuetta',
'@SarfarazA_54',
'💜❤️',
'very',
'well',
'played',
'@JasonRoy20…',
'RT',
'@CricMady:',
'Viv',
'Richards:',
'Lahore',
'Qalandars',
'have',
'the',
'best',
'bowling',
'attack',
'they',
'have',
'best',
'international',
'bowlers',
'but',
'it',
'was',
'a',
'fantastic',
'innin…',
'#JasonRoy',
'brilliant',
'entry',
'in',
'PSL',
'#PSL7',
'#LevelHai',
'https://t.co/KwuKudNgVu',
'Lahore',
'aane',
'do',
'ise',
'#jasonroy',
'#ShaheenShahAfridi',
'https://t.co/kITphBCE8w',
'#JasonRoy',
'Remember',
'when',
'this',
'happened?',
'🤯',
'It’s',
'Lala',
'vs',
'Shaheen',
'today.',
'#HBLPSL7',
'l',
'#shahidafridi',
'#QGvLQ',
'https://t.co/LXdWauCwS7',
'Excellent',
'display',
'of',
'batting',
'👌',
'#Jasonroy',
'روئے',
'آپ',
'کی',
'وجہ',
'سے',
'آج',
'لاہوری',
'بہت',
'روئے',
'ہیں',
'۔😉😉',
'Congratulations',
'@TeamQuetta',
'@SarfarazA_54',
'💜❤️',
'very',
'well',
'played…',
'https://t.co/wliIF8GWbq',
'Jason',
'Roy',
'https://t.co/tYG8PUe0Kj',
'#LQvQG',
'#QGvsLQ',
'#QGvLQ',
'#jasonroy',
'Quetta',
'Gladiators',
'batter',
'Jason',
'Roy',
'shined',
'the',
'brightest',
'on',
'Monday',
'as',
'he',
'sailed',
'his',
'team',
'to',
'a',
'seven-wicket',
'victory',
'a…',
'https://t.co/Oy1t0JQKDF',
'Excellent',
'batting',
'by',
'Jason',
'Roy',
'#JasonRoy',
'#Sarfraz',
'#ShaheenShahAfridi',
'#Shaheen',
'#QGvLQ',
'#LQvQG',
'#HBLPSL7',
'#HBLPSL2022',
'https://t.co/pDD2hMT0bA',
'Jason',
'Roy',
'to',
'Lahore',
'Qalandars:',
'#JASONROY',
'#QGvLQ',
'https://t.co/HedDvdzSqR',
'JASON',
'SUPER',
'SUPER',
'ROY',
'KA',
'#LevelHai',
'#Jasonroy',
'#PSL7',
'#PurpleForce',
'#QGvsLQ',
'#PSL2022',
'@JasonRoy20',
'@TeamQuetta',
'@iNabeelHashmi',
'@MichaelVaughan',
'@JasonRoy20',
'One',
'of',
'the',
'best',
'T20',
'innings,',
'I',
'have',
'had',
'witnessed',
'in',
'the',
'game.',
'#JasonRoy',
'@JasonRoy20',
'#PSL',
'#QGvLQ',
'RT',
'@Maliiihaarif:',
'Sahi',
'ly',
'rha',
'hai',
'Roy',
'Lahore',
'ki',
'bohat',
'mazak',
'bna',
'rhe',
'the',
'na',
'hum',
'Karachi',
'walo',
'ka.',
'#jasonRoy',
'#QGvsLQ',
'#HBLPSL7',
'Sarfaraz',
'Ahmed:',
'The',
'more',
'you',
'praise',
'this',
'innings,',
'the',
'lesser',
'it',
'would',
'be.',
'One',
'of',
'the',
'best',
'knocks',
'in',
'the',
'PSL.',
'We',
'pla…',
'https://t.co/xfiNL5I33U',
'RT',
'@partisanofKhan1:',
'Situation',
'after',
'#QGvsLQ',
'game!',
'😏',
'#HBLPSL7',
'#JasonRoy',
'#PurpleForce',
'https://t.co/GIxo0NiaIq',
'RT',
'@arynewsud:',
'’جیسن',
'روئے',
'نے',
'سرفراز',
'کے',
'چہرے',
'پر',
'مسکراہٹ',
'بکھیر',
'دی‘',
'قلندرز',
'کو',
'شکست',
'#ARYNewsUrdu',
'#QGvLQ',
'#JasonRoy',
'#SarfarazAhmed',
'#PSL7',
'https:/…',
'Quetta',
'Gladiators',
'won',
'by',
'7',
'wickets',
'#JasonRoy',
'#Sarfraz',
'#Shaheen',
'#ShaheenShahAfridi',
'#QGvLQ',
'#lqvsqg',
'#HBLPSL7',
'#HBLPSL2022',
'RT',
'@CricMady:',
'Jason',
'Roy,',
'Player',
'of',
'the',
'Match:',
'I',
'have',
'been',
'extremely',
'jet',
'lagged.',
'It',
'has',
'been',
'a',
'long',
'time',
'since',
'my',
'last',
'T20',
'hundred.',
'To',
'stri…',
'RT',
'@YStorai:',
'Congratulations',
'Quetta',
'Gladiators.',
'Lahoriyo',
'kesa',
'lg',
'rha',
'😂',
'3',
'se',
'4',
'match',
'kiya',
'jeety',
'sara',
'#Pakistan',
'sr',
'pr',
'uthaya',
'ta😅',
'#QGvLQ',
'Well…',
'@cricbuzz',
'Absolutely',
'Sensational',
'#QGvLQ',
'#psl72022',
'#JasonRoy',
'Mere',
'hathu',
'men',
'Bat',
'hai',
'koi',
'aslaa',
'tou',
'naii,',
'Chakkay',
'shakkay',
'maar',
'lu?',
'Koi',
'masla',
'tou',
'naii',
'!',
'#JasonRoy',
'😂',
'RT',
'@beggirlrizwan7:',
'@thePSLt20',
'@TeamQuetta',
'Aaj',
'laga',
'ki',
'koi',
'accha',
'match',
'dekha',
'psl',
'ka',
'#LQvQG',
'#Jasonroy',
'#PSL7',
'#PSL2022',
'RT',
'@NaikRooh:',
'It',
'takes',
'a',
'single',
'Gladiator',
'to',
'destroy',
'the',
'favorites...',
'#JasonRoy',
'https://t.co/9iIeqtjXOn',
'Jason',
'Roy',
'was',
'our',
'player',
'of',
'the',
'match',
'today',
'after',
'he',
'led',
'the',
'Gladiators',
'to',
'a',
'fantastic',
'victory',
'in',
'the',
'last',
'HBL',
'PSL…',
'https://t.co/IlJJelZKJF',
'RT',
'@ShezzyAwan:',
'گل',
'وڈ',
'گئی',
'ہے۔۔۔!!',
'#zardari',
'#Pervaizelahi',
'#jasonroy',
'https://t.co/BCwjRldIOc',
'11',
'Fours',
'8',
'Sixes',
'Jason',
'Roy',
'was',
'at',
'his',
'entertaining',
'best',
'tonight!',
'#PSL2022',
'#t20cricket',
'#psl',
'#pakistansuperleague…',
'https://t.co/F45XQJ51AQ',
'Dear',
'Lahore',
'qalandar',
'never',
'do',
'more',
'than',
'200.',
"It's",
'not',
'suitable',
'for',
'you.',
'#LahoreQalandars',
'#shaheen',
'#jasonroy',
'#QGvLQ',
'RT',
'@Abdullah_Editzz:',
'Fastest',
'Century',
'Of',
'PSL',
'7',
',',
'Destructive',
'Batting',
'From',
'Jason',
'Roy',
',',
'One',
'Of',
'The',
'Best',
'Inning',
'Of',
'PSL',
'🔥👑',
'#JasonRoy',
'||',
'#QGvLQ…',
'جیسن',
'روئے',
'کا',
'نیا',
'ریکارڈ؛',
'آفریدی',
'کو',
'لگایا',
'چھکا',
'یادگار',
'بن',
'گیا',
'#ARYNewsUrdu',
'#JasonRoy',
'#shahidafridi',
'https://t.co/YiwdvXyU06',
'RT',
'@khelshel:',
'No',
'surprise,',
'Jason',
'Roy',
'gets',
'player',
'of',
'the',
'match',
'award',
'for',
'registering',
'2nd',
'fastest',
'hundred',
'of',
'#PSL',
'history.',
'#Cricket',
'|',
'#Pakis…',
'Every',
'bowl',
'he',
'bowls',
'is',
'either',
'a',
'short',
'ball',
'or',
'a',
'full',
'toss,',
'that',
'too',
'with',
'a',
'lot',
'of',
'pace,',
'so',
'it',
'just',
'gives',
'the',
'batter…',
'https://t.co/Rl1fo8WoXZ',
'WEST:',
'AGHUWAH',
'KAR-0-DACAIT',
'GIRAFTAAR',
'|',
'C110NEWS',
'HD',
'|',
'REPORTED',
'BY',
'TAIMOOR',
'SHAKIL',
'#C110NEWS',
'#JasonRoy',
'#QGvsLQ',
'#LQvQG…',
'https://t.co/se86E74Z2H',
'#jasonroy',
'https://t.co/Z2iG5sGEzH',
'https://t.co/sJs3v9bjAe',
'RT',
'@khelshel:',
'No',
'surprise,',
'Jason',
'Roy',
'gets',
'player',
'of',
'the',
'match',
'award',
'for',
'registering',
'2nd',
'fastest',
'hundred',
'of',
'#PSL',
'history.',
'#Cricket',
'|',
'#Pakis…',
'RT',
'@ChaudhryAzhar3:',
'Jason',
'Roy',
'is',
'going',
'big',
'in',
'the',
'PSL',
'brilliant',
'knock',
'from',
'Roy',
'well',
'deserving',
'100*',
'#jasonRoy',
'#LQvsQG',
'https://t.co/5YNx2KNcIn',
'RT',
'@BaaghiTV:',
'HBL',
'PSL:',
'Jason',
'Roy’s',
'quickfire',
'116',
'gets',
'Quetta',
'Gladiators',
'over',
'finish',
'line',
'https://t.co/vfrZBVYjb1',
'#JasonRoy',
'HBL',
'PSL:',
'Jason',
'Roy’s',
'quickfire',
'116',
'gets',
'Quetta',
'Gladiators',
'over',
'finish',
'line',
'https://t.co/vfrZBVYjb1',
'#JasonRoy',
'No',
'surprise,',
'Jason',
'Roy',
'gets',
'player',
'of',
'the',
'match',
'award',
'for',
'registering',
'2nd',
'fastest',
'hundred',
'of',
'#PSL',
'history.…',
'https://t.co/vxWpaGpcy9',
'Lets',
'tell',
'the',
'sensational',
'shehryar',
'afridi',
'not',
'to',
'be',
'in',
'hurry.',
'Its',
'PSL',
'not',
'KPL',
'#QGvsLQ',
'#jasonroy',
'https://t.co/imCtEX2UD0',
'RT',
'@CricMady:',
'Jason',
'Roy,',
'Player',
'of',
'the',
'Match:',
'I',
'have',
'been',
'extremely',
'jet',
'lagged.',
'It',
'has',
'been',
'a',
'long',
'time',
'since',
'my',
'last',
'T20',
'hundred.',
'To',
'stri…',
'Start',
'playing',
'your',
'life',
'INNINGS',
'like',
'#jasonRoy',
'😊',
'.',
'https://t.co/FCEaomGtQK',
'.',
'#SK',
'#Skcookware',
'#kitchentools…',
'https://t.co/fBpRs6JN8D',
'Situation',
'after',
'#QGvsLQ',
'game!',
'😏',
'#HBLPSL7',
'#JasonRoy',
'#PurpleForce',
'https://t.co/GIxo0NiaIq',
'Jason',
"Roy's",
'heroics',
'helped',
'Quetta',
'Gladiators',
'thump',
'Lahore',
'Qalandars',
'by',
'7',
'wickets',
'in',
'the',
'15th',
'match',
'of',
'the',
'Pakistan…',
'https://t.co/zDWQrEUXYF',
'@thePSLt20',
'@TeamQuetta',
'Aaj',
'laga',
'ki',
'koi',
'accha',
'match',
'dekha',
'psl',
'ka',
'#LQvQG',
'#Jasonroy',
'#PSL7',
'#PSL2022',
'Jason',
'Roy',
'made',
'that',
'chase',
'look',
'very',
'very',
'easy',
'For',
'more',
'sports',
'news',
'please',
'visit',
'https://t.co/UClxBrbs62',
'#PSL2022…',
'https://t.co/uig7tgcxYJ',
'Second',
'victory',
'for',
'Quetta',
'Gladiators',
'in',
'#PSL7',
'courtesy',
'blistering',
'hundred',
'of',
'Jason',
'Roy.',
'.',
'.',
'@JasonRoy20…',
'https://t.co/wPRP69RGQK',
'RT',
'@CricMady:',
'Sarfaraz',
'Ahmed:',
'The',
'more',
'you',
'praise',
'this',
'innings,',
'the',
'lesser',
'it',
'would',
'be.',
'One',
'of',
'the',
'best',
'knocks',
'in',
'the',
'PSL.',
'We',
'planned',
'to',
'st…',
'Shaheen',
':',
'ajeeb',
'admi',
'hy',
'yra',
'hmari',
'bari',
'baray',
'larko',
'ko',
'khila',
'leta',
'hy',
'😂',
'#QGvLQ',
'#jasonroy',
'#PSL2022',
'#psl',
'#sarfrazahmad…',
'https://t.co/TGSFgSJYxZ',
'RT',
'@CricksLab:',
"'He",
'Came,',
'He',
'Saw,',
'He',
"Conquered'",
'@JasonRoy20',
'giving',
'high',
'hopes',
'to',
'the',
'Quetta',
'Gladiators',
'as',
'they',
'beat',
'Lahore',
'Qalandars',
'by',
'7…',
'#jasonroy',
'you',
'are',
'beauty',
'what',
'a',
'play',
'boss',
'amazing',
'@JasonRoy20',
'#PSL7',
'#JasonRoy',
'https://t.co/Vt9UybqRPm',
'One',
'man',
'army',
'#jasonroy',
'#lqvsqg',
'#Psl7',
'https://t.co/H2NSJzzxKD',
...]
from wordcloud import WordCloud, STOPWORDS
import matplotlib.pyplot as plt
import pandas as pa
stupid= ["hi",'bye']
columns=["word"]
pa.DataFrame(stupid, columns = columns)
stopwords = set(STOPWORDS)
wordcloud = WordCloud(width = 800, height = 800,
background_color ='white',
stopwords = stopwords,
min_font_size = 10).generate(update)
# plot the WordCloud image
plt.figure(figsize = (8, 8))
plt.imshow(wordcloud)
plt.axis("off")
plt.tight_layout(pad = 0)
#plt.show()
plt.savefig('img.png')
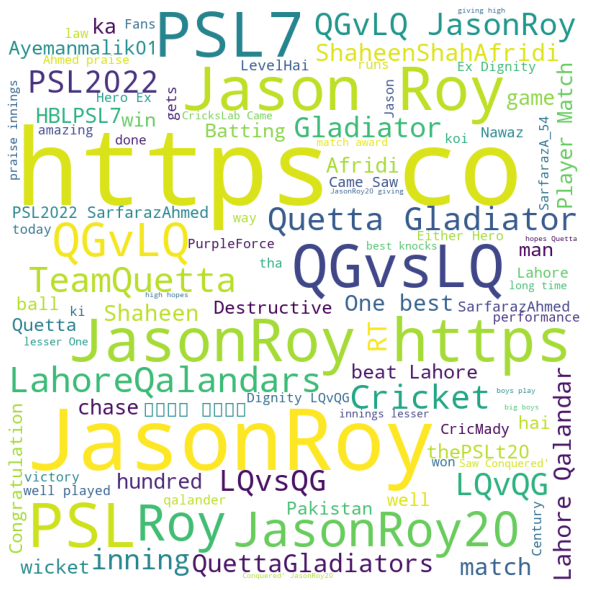
from PIL import Image
im = Image.open('img.png')
im
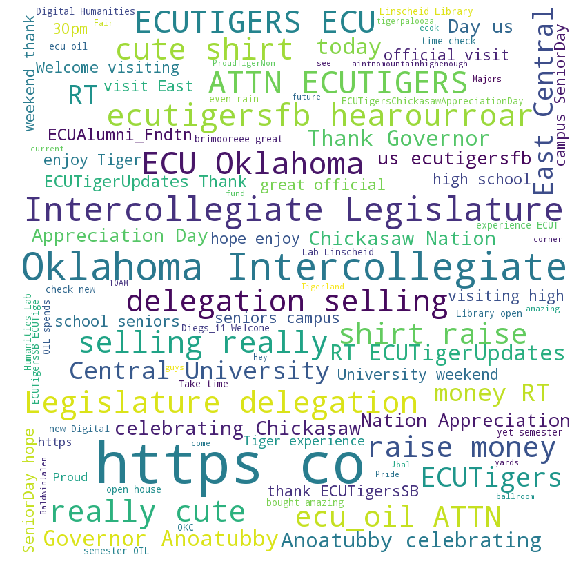
Using Prettytable#
from prettytable import PrettyTable
for label, data in (('Word', words),
('Screen Name', screen_names),
('Hashtag', hashtags)):
pt = PrettyTable(field_names=[label, 'Count'])
c = Counter(data)
[ pt.add_row(kv) for kv in c.most_common()[:10] ]
pt.align[label], pt.align['Count'] = 'l', 'r' # Set column alignment
print(pt)
+-----------+-------+
| Word | Count |
+-----------+-------+
| #JasonRoy | 86 |
| the | 64 |
| RT | 59 |
| a | 43 |
| Jason | 41 |
| #QGvsLQ | 34 |
| of | 34 |
| #jasonroy | 33 |
| in | 32 |
| #QGvLQ | 32 |
+-----------+-------+
+-----------------+-------+
| Screen Name | Count |
+-----------------+-------+
| JasonRoy20 | 29 |
| TeamQuetta | 18 |
| lahoreqalandars | 9 |
| Ayemanmalik01 | 7 |
| thePSLt20 | 7 |
| CricMady | 5 |
| SarfarazA_54 | 4 |
| CricksLab | 4 |
| arynewsud | 3 |
| NaikRooh | 3 |
+-----------------+-------+
+------------------+-------+
| Hashtag | Count |
+------------------+-------+
| JasonRoy | 87 |
| PSL7 | 37 |
| QGvsLQ | 36 |
| jasonroy | 34 |
| QGvLQ | 34 |
| PSL2022 | 22 |
| LQvQG | 20 |
| Cricket | 13 |
| Jasonroy | 11 |
| QuettaGladiators | 11 |
+------------------+-------+
Calculating Lexical Diversity#
# A function for computing lexical diversity
def lexical_diversity(tokens):
return 1.0*len(set(tokens))/len(tokens)
# A function for computing the average number of words per tweet
def average_words(statuses):
total_words = sum([ len(s.split()) for s in statuses ])
return 1.0*total_words/len(statuses)
print(lexical_diversity(words))
print(lexical_diversity(screen_names))
print(lexical_diversity(hashtags))
print(average_words(status_texts))
0.2688296639629201
0.2682926829268293
0.19047619047619047
19.177777777777777
Finding the most popular retweets#
retweets = [
# Store out a tuple of these three values ...
(status['retweet_count'],
status['retweeted_status']['user']['screen_name'],
status['text'])
# ... for each status ...
for status in statuses
# ... so long as the status meets this condition.
if 'retweeted_status' in status
]
# Slice off the first 5 from the sorted results and display each item in the tuple
pt = PrettyTable(field_names=['Count', 'Screen Name', 'Text'])
[ pt.add_row(row) for row in sorted(retweets, reverse=True)[:5] ]
pt.max_width['Text'] = 50
pt.align= 'l'
print(pt)
+-------+-------------+----------------------------------------------------+
| Count | Screen Name | Text |
+-------+-------------+----------------------------------------------------+
| 26 | msarosh | RT @msarosh: I think thats the best innings of the |
| | | Pakistan Super League ever. Its not just about |
| | | 100, the way he paced the innings phase w… |
| 8 | NaikRooh | RT @NaikRooh: It takes a single Gladiator to |
| | | destroy the favorites... #JasonRoy |
| | | https://t.co/9iIeqtjXOn |
| 8 | NaikRooh | RT @NaikRooh: It takes a single Gladiator to |
| | | destroy the favorites... #JasonRoy |
| | | https://t.co/9iIeqtjXOn |
| 8 | NaikRooh | RT @NaikRooh: It takes a single Gladiator to |
| | | destroy the favorites... #JasonRoy |
| | | https://t.co/9iIeqtjXOn |
| 7 | itx_Aehna | RT @itx_Aehna: Man of the moment #JasonRoy |
| | | #QGvPZ https://t.co/VktyjQ0VTk |
+-------+-------------+----------------------------------------------------+
Plotting frequencies of words#
import matplotlib
import matplotlib.pyplot as plt
%matplotlib inline
word_counts = sorted(Counter(words).values(), reverse=True)
plt.loglog(word_counts)
plt.ylabel("Freq")
plt.xlabel("Word Rank")
<matplotlib.text.Text at 0x7f0497be26a0>
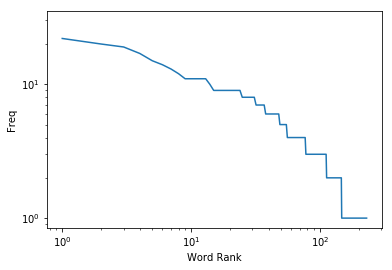
Posting It Back on the Web#
import datetime
now = datetime.datetime.now()
print(now)
2022-01-31 22:06:50.050192
popular = c.most_common()[0][0]
now = datetime.date(now.year,now.month,now.day)
print(now)
2018-10-16
mathcs_twitter_api.statuses.update(
status="#ECUTigers are talking about {} today on {} @cs_ecu".format(popular,now))
{'contributors': None,
'coordinates': None,
'created_at': 'Tue Oct 16 21:21:00 +0000 2018',
'entities': {'hashtags': [{'indices': [0, 10], 'text': 'ECUTigers'}],
'symbols': [],
'urls': [],
'user_mentions': [{'id': 1037047347405045760,
'id_str': '1037047347405045760',
'indices': [59, 66],
'name': 'ECU_Math/CS',
'screen_name': 'cs_ecu'}]},
'favorite_count': 0,
'favorited': False,
'geo': None,
'id': 1052308450976378880,
'id_str': '1052308450976378880',
'in_reply_to_screen_name': None,
'in_reply_to_status_id': None,
'in_reply_to_status_id_str': None,
'in_reply_to_user_id': None,
'in_reply_to_user_id_str': None,
'is_quote_status': False,
'lang': 'en',
'place': None,
'retweet_count': 0,
'retweeted': False,
'source': '<a href="http://ecok.edu/academics/colleges-and-schools/college-health-and-sciences/department-mathematics-and-computer" rel="nofollow">Starlas Data Project</a>',
'text': '#ECUTigers are talking about ECUTigers today on 2018-10-16 @cs_ecu',
'truncated': False,
'user': {'contributors_enabled': False,
'created_at': 'Tue Sep 04 18:38:49 +0000 2018',
'default_profile': False,
'default_profile_image': False,
'description': '',
'entities': {'description': {'urls': []},
'url': {'urls': [{'display_url': 'bit.ly/2NfSiTh',
'expanded_url': 'https://bit.ly/2NfSiTh',
'indices': [0, 23],
'url': 'https://t.co/PugruxKlLL'}]}},
'favourites_count': 0,
'follow_request_sent': False,
'followers_count': 5,
'following': False,
'friends_count': 29,
'geo_enabled': False,
'has_extended_profile': False,
'id': 1037047347405045760,
'id_str': '1037047347405045760',
'is_translation_enabled': False,
'is_translator': False,
'lang': 'en',
'listed_count': 0,
'location': 'Ada, OK',
'name': 'ECU_Math/CS',
'notifications': False,
'profile_background_color': '000000',
'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png',
'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png',
'profile_background_tile': False,
'profile_banner_url': 'https://pbs.twimg.com/profile_banners/1037047347405045760/1536096251',
'profile_image_url': 'http://pbs.twimg.com/profile_images/1037089960514310144/Kk2ypzpr_normal.jpg',
'profile_image_url_https': 'https://pbs.twimg.com/profile_images/1037089960514310144/Kk2ypzpr_normal.jpg',
'profile_link_color': 'FF5400',
'profile_sidebar_border_color': '000000',
'profile_sidebar_fill_color': '000000',
'profile_text_color': '000000',
'profile_use_background_image': False,
'protected': False,
'screen_name': 'cs_ecu',
'statuses_count': 6,
'time_zone': None,
'translator_type': 'none',
'url': 'https://t.co/PugruxKlLL',
'utc_offset': None,
'verified': False}}
mathcs_twitter_api.statuses.update(
status="#ECUTigers are talking about {} today on {} @cs_ecu".format(popular,now))
File "<ipython-input-73-4f32b376ab6a>", line 1
nurfnick_twitter_api.statuses.update_with_media("media[]":"im",
^
SyntaxError: invalid syntax
retweets = [
# Store out a tuple of these three values ...
(status['retweeted_status']['user']['screen_name'],
status['text'])
# ... for each status ...
for status in statuses
# ... so long as the status meets this condition.
if 'retweeted_status' in status
]
retweets[1]
('ECUTigerUpdates',
'RT @ECUTigerUpdates: Take time to check out the new Digital Humanities Lab in the Linscheid Library. The open house is today from 3-4:30pm…')
mathcs_twitter_api.statuses.update(
status="#ECUTigers are saying `{}` today on {} @cs_ecu".format(retweets[1],now))
{'contributors': None,
'coordinates': None,
'created_at': 'Tue Oct 16 21:29:54 +0000 2018',
'entities': {'hashtags': [{'indices': [0, 10], 'text': 'ECUTigers'}],
'symbols': [],
'urls': [{'display_url': 'twitter.com/i/web/status/1…',
'expanded_url': 'https://twitter.com/i/web/status/1052310693339439104',
'indices': [116, 139],
'url': 'https://t.co/Pp9R3Z0hMr'}],
'user_mentions': [{'id': 44617318,
'id_str': '44617318',
'indices': [47, 63],
'name': 'East Central Univ.',
'screen_name': 'ECUTigerUpdates'}]},
'favorite_count': 0,
'favorited': False,
'geo': None,
'id': 1052310693339439104,
'id_str': '1052310693339439104',
'in_reply_to_screen_name': None,
'in_reply_to_status_id': None,
'in_reply_to_status_id_str': None,
'in_reply_to_user_id': None,
'in_reply_to_user_id_str': None,
'is_quote_status': False,
'lang': 'en',
'place': None,
'retweet_count': 0,
'retweeted': False,
'source': '<a href="http://ecok.edu/academics/colleges-and-schools/college-health-and-sciences/department-mathematics-and-computer" rel="nofollow">Starlas Data Project</a>',
'text': "#ECUTigers are saying `('ECUTigerUpdates', 'RT @ECUTigerUpdates: Take time to check out the new Digital Humanities… https://t.co/Pp9R3Z0hMr",
'truncated': True,
'user': {'contributors_enabled': False,
'created_at': 'Tue Sep 04 18:38:49 +0000 2018',
'default_profile': False,
'default_profile_image': False,
'description': '',
'entities': {'description': {'urls': []},
'url': {'urls': [{'display_url': 'bit.ly/2NfSiTh',
'expanded_url': 'https://bit.ly/2NfSiTh',
'indices': [0, 23],
'url': 'https://t.co/PugruxKlLL'}]}},
'favourites_count': 0,
'follow_request_sent': False,
'followers_count': 5,
'following': False,
'friends_count': 29,
'geo_enabled': False,
'has_extended_profile': False,
'id': 1037047347405045760,
'id_str': '1037047347405045760',
'is_translation_enabled': False,
'is_translator': False,
'lang': 'en',
'listed_count': 0,
'location': 'Ada, OK',
'name': 'ECU_Math/CS',
'notifications': False,
'profile_background_color': '000000',
'profile_background_image_url': 'http://abs.twimg.com/images/themes/theme1/bg.png',
'profile_background_image_url_https': 'https://abs.twimg.com/images/themes/theme1/bg.png',
'profile_background_tile': False,
'profile_banner_url': 'https://pbs.twimg.com/profile_banners/1037047347405045760/1536096251',
'profile_image_url': 'http://pbs.twimg.com/profile_images/1037089960514310144/Kk2ypzpr_normal.jpg',
'profile_image_url_https': 'https://pbs.twimg.com/profile_images/1037089960514310144/Kk2ypzpr_normal.jpg',
'profile_link_color': 'FF5400',
'profile_sidebar_border_color': '000000',
'profile_sidebar_fill_color': '000000',
'profile_text_color': '000000',
'profile_use_background_image': False,
'protected': False,
'screen_name': 'cs_ecu',
'statuses_count': 8,
'time_zone': None,
'translator_type': 'none',
'url': 'https://t.co/PugruxKlLL',
'utc_offset': None,
'verified': False}}
Getting Home Pages and Past Tweets#
We can grab the home timeline from our user.
len(mathcs_twitter_api.statuses.home_timeline())
20
Or another user
len(mathcs_twitter_api.statuses.user_timeline(screen_name="nurfnick"))
20
I am going to guess here that the API is limiting me to only the past 20 tweets. You’ll find that there are lots of limits in the API!
Your Turn#
Using either the math_cs credentials or your own;
Get authenticated to twitter.
Find the top trending 10 topics in the world and display them by name
Search for your favorite show, actor, sports team or player and see what twitter is saying about them.